Proxmox Virtual Environment (Proxmox VE) v6.10.1 published on Friday, Jun 28, 2024 by Daniel Muehlbachler-Pietrzykowski
proxmoxve.Permission.getUser
Explore with Pulumi AI
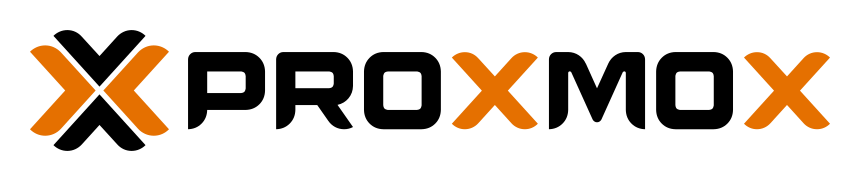
Proxmox Virtual Environment (Proxmox VE) v6.10.1 published on Friday, Jun 28, 2024 by Daniel Muehlbachler-Pietrzykowski
Retrieves information about a specific user.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@pulumi/proxmoxve";
const operationsUser = proxmoxve.Permission.getUser({
userId: "operation@pam",
});
import pulumi
import pulumi_proxmoxve as proxmoxve
operations_user = proxmoxve.Permission.get_user(user_id="operation@pam")
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/Permission"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := Permission.GetUser(ctx, &permission.GetUserArgs{
UserId: "operation@pam",
}, nil)
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var operationsUser = ProxmoxVE.Permission.GetUser.Invoke(new()
{
UserId = "operation@pam",
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Permission.PermissionFunctions;
import com.pulumi.proxmoxve.Permission.inputs.GetUserArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var operationsUser = PermissionFunctions.getUser(GetUserArgs.builder()
.userId("operation@pam")
.build());
}
}
variables:
operationsUser:
fn::invoke:
Function: proxmoxve:Permission:getUser
Arguments:
userId: operation@pam
Using getUser
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getUser(args: GetUserArgs, opts?: InvokeOptions): Promise<GetUserResult>
function getUserOutput(args: GetUserOutputArgs, opts?: InvokeOptions): Output<GetUserResult>
def get_user(user_id: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetUserResult
def get_user_output(user_id: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetUserResult]
func GetUser(ctx *Context, args *GetUserArgs, opts ...InvokeOption) (*GetUserResult, error)
func GetUserOutput(ctx *Context, args *GetUserOutputArgs, opts ...InvokeOption) GetUserResultOutput
> Note: This function is named GetUser
in the Go SDK.
public static class GetUser
{
public static Task<GetUserResult> InvokeAsync(GetUserArgs args, InvokeOptions? opts = null)
public static Output<GetUserResult> Invoke(GetUserInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetUserResult> getUser(GetUserArgs args, InvokeOptions options)
// Output-based functions aren't available in Java yet
fn::invoke:
function: proxmoxve:Permission/getUser:getUser
arguments:
# arguments dictionary
The following arguments are supported:
- User
Id string - The user identifier.
- User
Id string - The user identifier.
- user
Id String - The user identifier.
- user
Id string - The user identifier.
- user_
id str - The user identifier.
- user
Id String - The user identifier.
getUser Result
The following output properties are available:
- Acls
List<Pulumi.
Proxmox VE. Permission. Outputs. Get User Acl> - The access control list.
- Comment string
- The user comment.
- Email string
- The user's email address.
- Enabled bool
- Whether the user account is enabled.
- Expiration
Date string - The user account's expiration date (RFC 3339).
- First
Name string - The user's first name.
- Groups List<string>
- The user's groups.
- Id string
- The provider-assigned unique ID for this managed resource.
- Keys string
- The user's keys.
- Last
Name string - The user's last name.
- User
Id string
- Acls
[]Get
User Acl - The access control list.
- Comment string
- The user comment.
- Email string
- The user's email address.
- Enabled bool
- Whether the user account is enabled.
- Expiration
Date string - The user account's expiration date (RFC 3339).
- First
Name string - The user's first name.
- Groups []string
- The user's groups.
- Id string
- The provider-assigned unique ID for this managed resource.
- Keys string
- The user's keys.
- Last
Name string - The user's last name.
- User
Id string
- acls
List<Get
User Acl> - The access control list.
- comment String
- The user comment.
- email String
- The user's email address.
- enabled Boolean
- Whether the user account is enabled.
- expiration
Date String - The user account's expiration date (RFC 3339).
- first
Name String - The user's first name.
- groups List<String>
- The user's groups.
- id String
- The provider-assigned unique ID for this managed resource.
- keys String
- The user's keys.
- last
Name String - The user's last name.
- user
Id String
- acls
Get
User Acl[] - The access control list.
- comment string
- The user comment.
- email string
- The user's email address.
- enabled boolean
- Whether the user account is enabled.
- expiration
Date string - The user account's expiration date (RFC 3339).
- first
Name string - The user's first name.
- groups string[]
- The user's groups.
- id string
- The provider-assigned unique ID for this managed resource.
- keys string
- The user's keys.
- last
Name string - The user's last name.
- user
Id string
- acls
Sequence[permission.
Get User Acl] - The access control list.
- comment str
- The user comment.
- email str
- The user's email address.
- enabled bool
- Whether the user account is enabled.
- expiration_
date str - The user account's expiration date (RFC 3339).
- first_
name str - The user's first name.
- groups Sequence[str]
- The user's groups.
- id str
- The provider-assigned unique ID for this managed resource.
- keys str
- The user's keys.
- last_
name str - The user's last name.
- user_
id str
- acls List<Property Map>
- The access control list.
- comment String
- The user comment.
- email String
- The user's email address.
- enabled Boolean
- Whether the user account is enabled.
- expiration
Date String - The user account's expiration date (RFC 3339).
- first
Name String - The user's first name.
- groups List<String>
- The user's groups.
- id String
- The provider-assigned unique ID for this managed resource.
- keys String
- The user's keys.
- last
Name String - The user's last name.
- user
Id String
Supporting Types
GetUserAcl
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
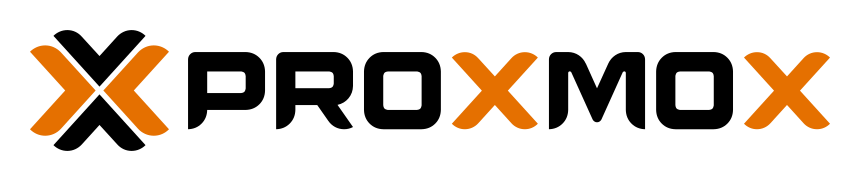
Proxmox Virtual Environment (Proxmox VE) v6.10.1 published on Friday, Jun 28, 2024 by Daniel Muehlbachler-Pietrzykowski