proxmoxve.Network.NetworkVlan
Explore with Pulumi AI
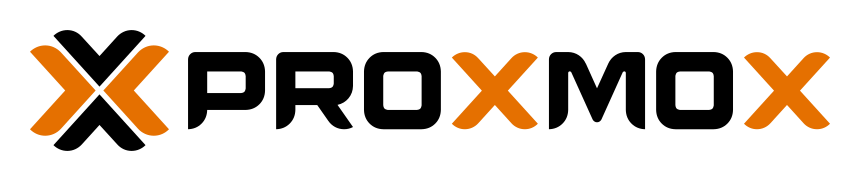
Manages a Linux VLAN network interface in a Proxmox VE node.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@muhlba91/pulumi-proxmoxve";
// using VLAN tag
const vlan99 = new proxmoxve.network.NetworkVlan("vlan99", {
comment: "VLAN 99",
nodeName: "pve",
});
// using custom network interface name
const vlan98 = new proxmoxve.network.NetworkVlan("vlan98", {
comment: "VLAN 98",
"interface": "eno0",
nodeName: "pve",
vlan: 98,
});
import pulumi
import pulumi_proxmoxve as proxmoxve
# using VLAN tag
vlan99 = proxmoxve.network.NetworkVlan("vlan99",
comment="VLAN 99",
node_name="pve")
# using custom network interface name
vlan98 = proxmoxve.network.NetworkVlan("vlan98",
comment="VLAN 98",
interface="eno0",
node_name="pve",
vlan=98)
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/Network"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
// using VLAN tag
_, err := Network.NewNetworkVlan(ctx, "vlan99", &Network.NetworkVlanArgs{
Comment: pulumi.String("VLAN 99"),
NodeName: pulumi.String("pve"),
})
if err != nil {
return err
}
// using custom network interface name
_, err = Network.NewNetworkVlan(ctx, "vlan98", &Network.NetworkVlanArgs{
Comment: pulumi.String("VLAN 98"),
Interface: pulumi.String("eno0"),
NodeName: pulumi.String("pve"),
Vlan: pulumi.Int(98),
})
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
// using VLAN tag
var vlan99 = new ProxmoxVE.Network.NetworkVlan("vlan99", new()
{
Comment = "VLAN 99",
NodeName = "pve",
});
// using custom network interface name
var vlan98 = new ProxmoxVE.Network.NetworkVlan("vlan98", new()
{
Comment = "VLAN 98",
Interface = "eno0",
NodeName = "pve",
Vlan = 98,
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Network.NetworkVlan;
import com.pulumi.proxmoxve.Network.NetworkVlanArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
// using VLAN tag
var vlan99 = new NetworkVlan("vlan99", NetworkVlanArgs.builder()
.comment("VLAN 99")
.nodeName("pve")
.build());
// using custom network interface name
var vlan98 = new NetworkVlan("vlan98", NetworkVlanArgs.builder()
.comment("VLAN 98")
.interface_("eno0")
.nodeName("pve")
.vlan(98)
.build());
}
}
resources:
# using VLAN tag
vlan99:
type: proxmoxve:Network:NetworkVlan
properties:
comment: VLAN 99
nodeName: pve
# using custom network interface name
vlan98:
type: proxmoxve:Network:NetworkVlan
properties:
comment: VLAN 98
interface: eno0
nodeName: pve
vlan: 98
Create NetworkVlan Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new NetworkVlan(name: string, args: NetworkVlanArgs, opts?: CustomResourceOptions);
@overload
def NetworkVlan(resource_name: str,
args: NetworkVlanArgs,
opts: Optional[ResourceOptions] = None)
@overload
def NetworkVlan(resource_name: str,
opts: Optional[ResourceOptions] = None,
node_name: Optional[str] = None,
address: Optional[str] = None,
address6: Optional[str] = None,
autostart: Optional[bool] = None,
comment: Optional[str] = None,
gateway: Optional[str] = None,
gateway6: Optional[str] = None,
interface: Optional[str] = None,
mtu: Optional[int] = None,
name: Optional[str] = None,
vlan: Optional[int] = None)
func NewNetworkVlan(ctx *Context, name string, args NetworkVlanArgs, opts ...ResourceOption) (*NetworkVlan, error)
public NetworkVlan(string name, NetworkVlanArgs args, CustomResourceOptions? opts = null)
public NetworkVlan(String name, NetworkVlanArgs args)
public NetworkVlan(String name, NetworkVlanArgs args, CustomResourceOptions options)
type: proxmoxve:Network:NetworkVlan
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args NetworkVlanArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args NetworkVlanArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args NetworkVlanArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args NetworkVlanArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args NetworkVlanArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var networkVlanResource = new ProxmoxVE.Network.NetworkVlan("networkVlanResource", new()
{
NodeName = "string",
Address = "string",
Address6 = "string",
Autostart = false,
Comment = "string",
Gateway = "string",
Gateway6 = "string",
Interface = "string",
Mtu = 0,
Name = "string",
Vlan = 0,
});
example, err := Network.NewNetworkVlan(ctx, "networkVlanResource", &Network.NetworkVlanArgs{
NodeName: pulumi.String("string"),
Address: pulumi.String("string"),
Address6: pulumi.String("string"),
Autostart: pulumi.Bool(false),
Comment: pulumi.String("string"),
Gateway: pulumi.String("string"),
Gateway6: pulumi.String("string"),
Interface: pulumi.String("string"),
Mtu: pulumi.Int(0),
Name: pulumi.String("string"),
Vlan: pulumi.Int(0),
})
var networkVlanResource = new NetworkVlan("networkVlanResource", NetworkVlanArgs.builder()
.nodeName("string")
.address("string")
.address6("string")
.autostart(false)
.comment("string")
.gateway("string")
.gateway6("string")
.interface_("string")
.mtu(0)
.name("string")
.vlan(0)
.build());
network_vlan_resource = proxmoxve.network.NetworkVlan("networkVlanResource",
node_name="string",
address="string",
address6="string",
autostart=False,
comment="string",
gateway="string",
gateway6="string",
interface="string",
mtu=0,
name="string",
vlan=0)
const networkVlanResource = new proxmoxve.network.NetworkVlan("networkVlanResource", {
nodeName: "string",
address: "string",
address6: "string",
autostart: false,
comment: "string",
gateway: "string",
gateway6: "string",
"interface": "string",
mtu: 0,
name: "string",
vlan: 0,
});
type: proxmoxve:Network:NetworkVlan
properties:
address: string
address6: string
autostart: false
comment: string
gateway: string
gateway6: string
interface: string
mtu: 0
name: string
nodeName: string
vlan: 0
NetworkVlan Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The NetworkVlan resource accepts the following input properties:
- Node
Name string - The name of the node.
- Address string
- The interface IPv4/CIDR address.
- Address6 string
- The interface IPv6/CIDR address.
- Autostart bool
- Automatically start interface on boot (defaults to
true
). - Comment string
- Comment for the interface.
- Gateway string
- Default gateway address.
- Gateway6 string
- Default IPv6 gateway address.
- Interface string
- The VLAN raw device. See also
name
. - Mtu int
- The interface MTU.
- Name string
- The interface name. Either add the VLAN tag number to an existing interface name, e.g.
ens18.21
(and do not setinterface
andvlan
), or use custom name, e.g.vlan_lab
(interface
andvlan
are then required). - Vlan int
- The VLAN tag. See also
name
.
- Node
Name string - The name of the node.
- Address string
- The interface IPv4/CIDR address.
- Address6 string
- The interface IPv6/CIDR address.
- Autostart bool
- Automatically start interface on boot (defaults to
true
). - Comment string
- Comment for the interface.
- Gateway string
- Default gateway address.
- Gateway6 string
- Default IPv6 gateway address.
- Interface string
- The VLAN raw device. See also
name
. - Mtu int
- The interface MTU.
- Name string
- The interface name. Either add the VLAN tag number to an existing interface name, e.g.
ens18.21
(and do not setinterface
andvlan
), or use custom name, e.g.vlan_lab
(interface
andvlan
are then required). - Vlan int
- The VLAN tag. See also
name
.
- node
Name String - The name of the node.
- address String
- The interface IPv4/CIDR address.
- address6 String
- The interface IPv6/CIDR address.
- autostart Boolean
- Automatically start interface on boot (defaults to
true
). - comment String
- Comment for the interface.
- gateway String
- Default gateway address.
- gateway6 String
- Default IPv6 gateway address.
- interface_ String
- The VLAN raw device. See also
name
. - mtu Integer
- The interface MTU.
- name String
- The interface name. Either add the VLAN tag number to an existing interface name, e.g.
ens18.21
(and do not setinterface
andvlan
), or use custom name, e.g.vlan_lab
(interface
andvlan
are then required). - vlan Integer
- The VLAN tag. See also
name
.
- node
Name string - The name of the node.
- address string
- The interface IPv4/CIDR address.
- address6 string
- The interface IPv6/CIDR address.
- autostart boolean
- Automatically start interface on boot (defaults to
true
). - comment string
- Comment for the interface.
- gateway string
- Default gateway address.
- gateway6 string
- Default IPv6 gateway address.
- interface string
- The VLAN raw device. See also
name
. - mtu number
- The interface MTU.
- name string
- The interface name. Either add the VLAN tag number to an existing interface name, e.g.
ens18.21
(and do not setinterface
andvlan
), or use custom name, e.g.vlan_lab
(interface
andvlan
are then required). - vlan number
- The VLAN tag. See also
name
.
- node_
name str - The name of the node.
- address str
- The interface IPv4/CIDR address.
- address6 str
- The interface IPv6/CIDR address.
- autostart bool
- Automatically start interface on boot (defaults to
true
). - comment str
- Comment for the interface.
- gateway str
- Default gateway address.
- gateway6 str
- Default IPv6 gateway address.
- interface str
- The VLAN raw device. See also
name
. - mtu int
- The interface MTU.
- name str
- The interface name. Either add the VLAN tag number to an existing interface name, e.g.
ens18.21
(and do not setinterface
andvlan
), or use custom name, e.g.vlan_lab
(interface
andvlan
are then required). - vlan int
- The VLAN tag. See also
name
.
- node
Name String - The name of the node.
- address String
- The interface IPv4/CIDR address.
- address6 String
- The interface IPv6/CIDR address.
- autostart Boolean
- Automatically start interface on boot (defaults to
true
). - comment String
- Comment for the interface.
- gateway String
- Default gateway address.
- gateway6 String
- Default IPv6 gateway address.
- interface String
- The VLAN raw device. See also
name
. - mtu Number
- The interface MTU.
- name String
- The interface name. Either add the VLAN tag number to an existing interface name, e.g.
ens18.21
(and do not setinterface
andvlan
), or use custom name, e.g.vlan_lab
(interface
andvlan
are then required). - vlan Number
- The VLAN tag. See also
name
.
Outputs
All input properties are implicitly available as output properties. Additionally, the NetworkVlan resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing NetworkVlan Resource
Get an existing NetworkVlan resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: NetworkVlanState, opts?: CustomResourceOptions): NetworkVlan
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
address: Optional[str] = None,
address6: Optional[str] = None,
autostart: Optional[bool] = None,
comment: Optional[str] = None,
gateway: Optional[str] = None,
gateway6: Optional[str] = None,
interface: Optional[str] = None,
mtu: Optional[int] = None,
name: Optional[str] = None,
node_name: Optional[str] = None,
vlan: Optional[int] = None) -> NetworkVlan
func GetNetworkVlan(ctx *Context, name string, id IDInput, state *NetworkVlanState, opts ...ResourceOption) (*NetworkVlan, error)
public static NetworkVlan Get(string name, Input<string> id, NetworkVlanState? state, CustomResourceOptions? opts = null)
public static NetworkVlan get(String name, Output<String> id, NetworkVlanState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Address string
- The interface IPv4/CIDR address.
- Address6 string
- The interface IPv6/CIDR address.
- Autostart bool
- Automatically start interface on boot (defaults to
true
). - Comment string
- Comment for the interface.
- Gateway string
- Default gateway address.
- Gateway6 string
- Default IPv6 gateway address.
- Interface string
- The VLAN raw device. See also
name
. - Mtu int
- The interface MTU.
- Name string
- The interface name. Either add the VLAN tag number to an existing interface name, e.g.
ens18.21
(and do not setinterface
andvlan
), or use custom name, e.g.vlan_lab
(interface
andvlan
are then required). - Node
Name string - The name of the node.
- Vlan int
- The VLAN tag. See also
name
.
- Address string
- The interface IPv4/CIDR address.
- Address6 string
- The interface IPv6/CIDR address.
- Autostart bool
- Automatically start interface on boot (defaults to
true
). - Comment string
- Comment for the interface.
- Gateway string
- Default gateway address.
- Gateway6 string
- Default IPv6 gateway address.
- Interface string
- The VLAN raw device. See also
name
. - Mtu int
- The interface MTU.
- Name string
- The interface name. Either add the VLAN tag number to an existing interface name, e.g.
ens18.21
(and do not setinterface
andvlan
), or use custom name, e.g.vlan_lab
(interface
andvlan
are then required). - Node
Name string - The name of the node.
- Vlan int
- The VLAN tag. See also
name
.
- address String
- The interface IPv4/CIDR address.
- address6 String
- The interface IPv6/CIDR address.
- autostart Boolean
- Automatically start interface on boot (defaults to
true
). - comment String
- Comment for the interface.
- gateway String
- Default gateway address.
- gateway6 String
- Default IPv6 gateway address.
- interface_ String
- The VLAN raw device. See also
name
. - mtu Integer
- The interface MTU.
- name String
- The interface name. Either add the VLAN tag number to an existing interface name, e.g.
ens18.21
(and do not setinterface
andvlan
), or use custom name, e.g.vlan_lab
(interface
andvlan
are then required). - node
Name String - The name of the node.
- vlan Integer
- The VLAN tag. See also
name
.
- address string
- The interface IPv4/CIDR address.
- address6 string
- The interface IPv6/CIDR address.
- autostart boolean
- Automatically start interface on boot (defaults to
true
). - comment string
- Comment for the interface.
- gateway string
- Default gateway address.
- gateway6 string
- Default IPv6 gateway address.
- interface string
- The VLAN raw device. See also
name
. - mtu number
- The interface MTU.
- name string
- The interface name. Either add the VLAN tag number to an existing interface name, e.g.
ens18.21
(and do not setinterface
andvlan
), or use custom name, e.g.vlan_lab
(interface
andvlan
are then required). - node
Name string - The name of the node.
- vlan number
- The VLAN tag. See also
name
.
- address str
- The interface IPv4/CIDR address.
- address6 str
- The interface IPv6/CIDR address.
- autostart bool
- Automatically start interface on boot (defaults to
true
). - comment str
- Comment for the interface.
- gateway str
- Default gateway address.
- gateway6 str
- Default IPv6 gateway address.
- interface str
- The VLAN raw device. See also
name
. - mtu int
- The interface MTU.
- name str
- The interface name. Either add the VLAN tag number to an existing interface name, e.g.
ens18.21
(and do not setinterface
andvlan
), or use custom name, e.g.vlan_lab
(interface
andvlan
are then required). - node_
name str - The name of the node.
- vlan int
- The VLAN tag. See also
name
.
- address String
- The interface IPv4/CIDR address.
- address6 String
- The interface IPv6/CIDR address.
- autostart Boolean
- Automatically start interface on boot (defaults to
true
). - comment String
- Comment for the interface.
- gateway String
- Default gateway address.
- gateway6 String
- Default IPv6 gateway address.
- interface String
- The VLAN raw device. See also
name
. - mtu Number
- The interface MTU.
- name String
- The interface name. Either add the VLAN tag number to an existing interface name, e.g.
ens18.21
(and do not setinterface
andvlan
), or use custom name, e.g.vlan_lab
(interface
andvlan
are then required). - node
Name String - The name of the node.
- vlan Number
- The VLAN tag. See also
name
.
Import
#!/usr/bin/env sh
#Interfaces can be imported using the node_name:iface
format, e.g.
$ pulumi import proxmoxve:Network/networkVlan:NetworkVlan vlan99 pve:vlan99
To learn more about importing existing cloud resources, see Importing resources.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
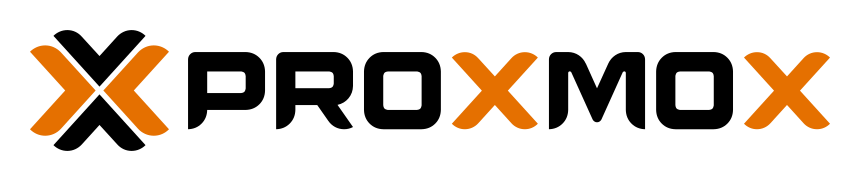