proxmoxve.Network.FirewallOptions
Explore with Pulumi AI
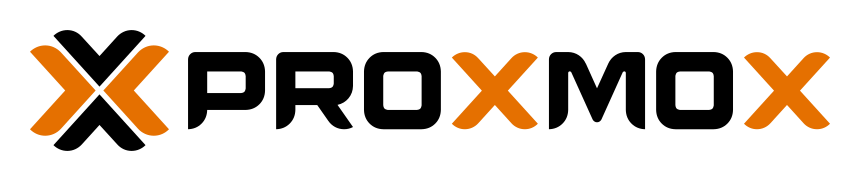
Manages firewall options on VM / Container level.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@muhlba91/pulumi-proxmoxve";
const example = new proxmoxve.network.FirewallOptions("example", {
nodeName: proxmox_virtual_environment_vm.example.node_name,
vmId: proxmox_virtual_environment_vm.example.vm_id,
dhcp: true,
enabled: false,
ipfilter: true,
logLevelIn: "info",
logLevelOut: "info",
macfilter: false,
ndp: true,
inputPolicy: "ACCEPT",
outputPolicy: "ACCEPT",
radv: true,
}, {
dependsOn: [proxmox_virtual_environment_vm.example],
});
import pulumi
import pulumi_proxmoxve as proxmoxve
example = proxmoxve.network.FirewallOptions("example",
node_name=proxmox_virtual_environment_vm["example"]["node_name"],
vm_id=proxmox_virtual_environment_vm["example"]["vm_id"],
dhcp=True,
enabled=False,
ipfilter=True,
log_level_in="info",
log_level_out="info",
macfilter=False,
ndp=True,
input_policy="ACCEPT",
output_policy="ACCEPT",
radv=True,
opts = pulumi.ResourceOptions(depends_on=[proxmox_virtual_environment_vm["example"]]))
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/Network"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := Network.NewFirewallOptions(ctx, "example", &Network.FirewallOptionsArgs{
NodeName: pulumi.Any(proxmox_virtual_environment_vm.Example.Node_name),
VmId: pulumi.Any(proxmox_virtual_environment_vm.Example.Vm_id),
Dhcp: pulumi.Bool(true),
Enabled: pulumi.Bool(false),
Ipfilter: pulumi.Bool(true),
LogLevelIn: pulumi.String("info"),
LogLevelOut: pulumi.String("info"),
Macfilter: pulumi.Bool(false),
Ndp: pulumi.Bool(true),
InputPolicy: pulumi.String("ACCEPT"),
OutputPolicy: pulumi.String("ACCEPT"),
Radv: pulumi.Bool(true),
}, pulumi.DependsOn([]pulumi.Resource{
proxmox_virtual_environment_vm.Example,
}))
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var example = new ProxmoxVE.Network.FirewallOptions("example", new()
{
NodeName = proxmox_virtual_environment_vm.Example.Node_name,
VmId = proxmox_virtual_environment_vm.Example.Vm_id,
Dhcp = true,
Enabled = false,
Ipfilter = true,
LogLevelIn = "info",
LogLevelOut = "info",
Macfilter = false,
Ndp = true,
InputPolicy = "ACCEPT",
OutputPolicy = "ACCEPT",
Radv = true,
}, new CustomResourceOptions
{
DependsOn =
{
proxmox_virtual_environment_vm.Example,
},
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Network.FirewallOptions;
import com.pulumi.proxmoxve.Network.FirewallOptionsArgs;
import com.pulumi.resources.CustomResourceOptions;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var example = new FirewallOptions("example", FirewallOptionsArgs.builder()
.nodeName(proxmox_virtual_environment_vm.example().node_name())
.vmId(proxmox_virtual_environment_vm.example().vm_id())
.dhcp(true)
.enabled(false)
.ipfilter(true)
.logLevelIn("info")
.logLevelOut("info")
.macfilter(false)
.ndp(true)
.inputPolicy("ACCEPT")
.outputPolicy("ACCEPT")
.radv(true)
.build(), CustomResourceOptions.builder()
.dependsOn(proxmox_virtual_environment_vm.example())
.build());
}
}
resources:
example:
type: proxmoxve:Network:FirewallOptions
properties:
nodeName: ${proxmox_virtual_environment_vm.example.node_name}
vmId: ${proxmox_virtual_environment_vm.example.vm_id}
dhcp: true
enabled: false
ipfilter: true
logLevelIn: info
logLevelOut: info
macfilter: false
ndp: true
inputPolicy: ACCEPT
outputPolicy: ACCEPT
radv: true
options:
dependson:
- ${proxmox_virtual_environment_vm.example}
Create FirewallOptions Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new FirewallOptions(name: string, args: FirewallOptionsArgs, opts?: CustomResourceOptions);
@overload
def FirewallOptions(resource_name: str,
args: FirewallOptionsArgs,
opts: Optional[ResourceOptions] = None)
@overload
def FirewallOptions(resource_name: str,
opts: Optional[ResourceOptions] = None,
node_name: Optional[str] = None,
input_policy: Optional[str] = None,
enabled: Optional[bool] = None,
container_id: Optional[int] = None,
ipfilter: Optional[bool] = None,
log_level_in: Optional[str] = None,
log_level_out: Optional[str] = None,
macfilter: Optional[bool] = None,
ndp: Optional[bool] = None,
dhcp: Optional[bool] = None,
output_policy: Optional[str] = None,
radv: Optional[bool] = None,
vm_id: Optional[int] = None)
func NewFirewallOptions(ctx *Context, name string, args FirewallOptionsArgs, opts ...ResourceOption) (*FirewallOptions, error)
public FirewallOptions(string name, FirewallOptionsArgs args, CustomResourceOptions? opts = null)
public FirewallOptions(String name, FirewallOptionsArgs args)
public FirewallOptions(String name, FirewallOptionsArgs args, CustomResourceOptions options)
type: proxmoxve:Network:FirewallOptions
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args FirewallOptionsArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args FirewallOptionsArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args FirewallOptionsArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args FirewallOptionsArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args FirewallOptionsArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var firewallOptionsResource = new ProxmoxVE.Network.FirewallOptions("firewallOptionsResource", new()
{
NodeName = "string",
InputPolicy = "string",
Enabled = false,
ContainerId = 0,
Ipfilter = false,
LogLevelIn = "string",
LogLevelOut = "string",
Macfilter = false,
Ndp = false,
Dhcp = false,
OutputPolicy = "string",
Radv = false,
VmId = 0,
});
example, err := Network.NewFirewallOptions(ctx, "firewallOptionsResource", &Network.FirewallOptionsArgs{
NodeName: pulumi.String("string"),
InputPolicy: pulumi.String("string"),
Enabled: pulumi.Bool(false),
ContainerId: pulumi.Int(0),
Ipfilter: pulumi.Bool(false),
LogLevelIn: pulumi.String("string"),
LogLevelOut: pulumi.String("string"),
Macfilter: pulumi.Bool(false),
Ndp: pulumi.Bool(false),
Dhcp: pulumi.Bool(false),
OutputPolicy: pulumi.String("string"),
Radv: pulumi.Bool(false),
VmId: pulumi.Int(0),
})
var firewallOptionsResource = new FirewallOptions("firewallOptionsResource", FirewallOptionsArgs.builder()
.nodeName("string")
.inputPolicy("string")
.enabled(false)
.containerId(0)
.ipfilter(false)
.logLevelIn("string")
.logLevelOut("string")
.macfilter(false)
.ndp(false)
.dhcp(false)
.outputPolicy("string")
.radv(false)
.vmId(0)
.build());
firewall_options_resource = proxmoxve.network.FirewallOptions("firewallOptionsResource",
node_name="string",
input_policy="string",
enabled=False,
container_id=0,
ipfilter=False,
log_level_in="string",
log_level_out="string",
macfilter=False,
ndp=False,
dhcp=False,
output_policy="string",
radv=False,
vm_id=0)
const firewallOptionsResource = new proxmoxve.network.FirewallOptions("firewallOptionsResource", {
nodeName: "string",
inputPolicy: "string",
enabled: false,
containerId: 0,
ipfilter: false,
logLevelIn: "string",
logLevelOut: "string",
macfilter: false,
ndp: false,
dhcp: false,
outputPolicy: "string",
radv: false,
vmId: 0,
});
type: proxmoxve:Network:FirewallOptions
properties:
containerId: 0
dhcp: false
enabled: false
inputPolicy: string
ipfilter: false
logLevelIn: string
logLevelOut: string
macfilter: false
ndp: false
nodeName: string
outputPolicy: string
radv: false
vmId: 0
FirewallOptions Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The FirewallOptions resource accepts the following input properties:
- Node
Name string - Node name.
- Container
Id int - Container ID. Leave empty for cluster level aliases.
- Dhcp bool
- Enable DHCP.
- Enabled bool
- Enable or disable the firewall.
- Input
Policy string - The default input
policy (
ACCEPT
,DROP
,REJECT
). - Ipfilter bool
- Enable default IP filters. This is equivalent to
adding an empty
ipfilter-net<id>
ipset for every interface. Such ipsets implicitly contain sane default restrictions such as restricting IPv6 link local addresses to the one derived from the interface's MAC address. For containers the configured IP addresses will be implicitly added. - Log
Level stringIn - Log level for incoming
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - Log
Level stringOut - Log level for outgoing
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - Macfilter bool
- Enable/disable MAC address filter.
- Ndp bool
- Enable NDP (Neighbor Discovery Protocol).
- Output
Policy string - The default output
policy (
ACCEPT
,DROP
,REJECT
). - Radv bool
- Enable Router Advertisement.
- Vm
Id int - VM ID. Leave empty for cluster level aliases.
- Node
Name string - Node name.
- Container
Id int - Container ID. Leave empty for cluster level aliases.
- Dhcp bool
- Enable DHCP.
- Enabled bool
- Enable or disable the firewall.
- Input
Policy string - The default input
policy (
ACCEPT
,DROP
,REJECT
). - Ipfilter bool
- Enable default IP filters. This is equivalent to
adding an empty
ipfilter-net<id>
ipset for every interface. Such ipsets implicitly contain sane default restrictions such as restricting IPv6 link local addresses to the one derived from the interface's MAC address. For containers the configured IP addresses will be implicitly added. - Log
Level stringIn - Log level for incoming
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - Log
Level stringOut - Log level for outgoing
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - Macfilter bool
- Enable/disable MAC address filter.
- Ndp bool
- Enable NDP (Neighbor Discovery Protocol).
- Output
Policy string - The default output
policy (
ACCEPT
,DROP
,REJECT
). - Radv bool
- Enable Router Advertisement.
- Vm
Id int - VM ID. Leave empty for cluster level aliases.
- node
Name String - Node name.
- container
Id Integer - Container ID. Leave empty for cluster level aliases.
- dhcp Boolean
- Enable DHCP.
- enabled Boolean
- Enable or disable the firewall.
- input
Policy String - The default input
policy (
ACCEPT
,DROP
,REJECT
). - ipfilter Boolean
- Enable default IP filters. This is equivalent to
adding an empty
ipfilter-net<id>
ipset for every interface. Such ipsets implicitly contain sane default restrictions such as restricting IPv6 link local addresses to the one derived from the interface's MAC address. For containers the configured IP addresses will be implicitly added. - log
Level StringIn - Log level for incoming
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - log
Level StringOut - Log level for outgoing
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - macfilter Boolean
- Enable/disable MAC address filter.
- ndp Boolean
- Enable NDP (Neighbor Discovery Protocol).
- output
Policy String - The default output
policy (
ACCEPT
,DROP
,REJECT
). - radv Boolean
- Enable Router Advertisement.
- vm
Id Integer - VM ID. Leave empty for cluster level aliases.
- node
Name string - Node name.
- container
Id number - Container ID. Leave empty for cluster level aliases.
- dhcp boolean
- Enable DHCP.
- enabled boolean
- Enable or disable the firewall.
- input
Policy string - The default input
policy (
ACCEPT
,DROP
,REJECT
). - ipfilter boolean
- Enable default IP filters. This is equivalent to
adding an empty
ipfilter-net<id>
ipset for every interface. Such ipsets implicitly contain sane default restrictions such as restricting IPv6 link local addresses to the one derived from the interface's MAC address. For containers the configured IP addresses will be implicitly added. - log
Level stringIn - Log level for incoming
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - log
Level stringOut - Log level for outgoing
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - macfilter boolean
- Enable/disable MAC address filter.
- ndp boolean
- Enable NDP (Neighbor Discovery Protocol).
- output
Policy string - The default output
policy (
ACCEPT
,DROP
,REJECT
). - radv boolean
- Enable Router Advertisement.
- vm
Id number - VM ID. Leave empty for cluster level aliases.
- node_
name str - Node name.
- container_
id int - Container ID. Leave empty for cluster level aliases.
- dhcp bool
- Enable DHCP.
- enabled bool
- Enable or disable the firewall.
- input_
policy str - The default input
policy (
ACCEPT
,DROP
,REJECT
). - ipfilter bool
- Enable default IP filters. This is equivalent to
adding an empty
ipfilter-net<id>
ipset for every interface. Such ipsets implicitly contain sane default restrictions such as restricting IPv6 link local addresses to the one derived from the interface's MAC address. For containers the configured IP addresses will be implicitly added. - log_
level_ strin - Log level for incoming
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - log_
level_ strout - Log level for outgoing
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - macfilter bool
- Enable/disable MAC address filter.
- ndp bool
- Enable NDP (Neighbor Discovery Protocol).
- output_
policy str - The default output
policy (
ACCEPT
,DROP
,REJECT
). - radv bool
- Enable Router Advertisement.
- vm_
id int - VM ID. Leave empty for cluster level aliases.
- node
Name String - Node name.
- container
Id Number - Container ID. Leave empty for cluster level aliases.
- dhcp Boolean
- Enable DHCP.
- enabled Boolean
- Enable or disable the firewall.
- input
Policy String - The default input
policy (
ACCEPT
,DROP
,REJECT
). - ipfilter Boolean
- Enable default IP filters. This is equivalent to
adding an empty
ipfilter-net<id>
ipset for every interface. Such ipsets implicitly contain sane default restrictions such as restricting IPv6 link local addresses to the one derived from the interface's MAC address. For containers the configured IP addresses will be implicitly added. - log
Level StringIn - Log level for incoming
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - log
Level StringOut - Log level for outgoing
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - macfilter Boolean
- Enable/disable MAC address filter.
- ndp Boolean
- Enable NDP (Neighbor Discovery Protocol).
- output
Policy String - The default output
policy (
ACCEPT
,DROP
,REJECT
). - radv Boolean
- Enable Router Advertisement.
- vm
Id Number - VM ID. Leave empty for cluster level aliases.
Outputs
All input properties are implicitly available as output properties. Additionally, the FirewallOptions resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing FirewallOptions Resource
Get an existing FirewallOptions resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: FirewallOptionsState, opts?: CustomResourceOptions): FirewallOptions
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
container_id: Optional[int] = None,
dhcp: Optional[bool] = None,
enabled: Optional[bool] = None,
input_policy: Optional[str] = None,
ipfilter: Optional[bool] = None,
log_level_in: Optional[str] = None,
log_level_out: Optional[str] = None,
macfilter: Optional[bool] = None,
ndp: Optional[bool] = None,
node_name: Optional[str] = None,
output_policy: Optional[str] = None,
radv: Optional[bool] = None,
vm_id: Optional[int] = None) -> FirewallOptions
func GetFirewallOptions(ctx *Context, name string, id IDInput, state *FirewallOptionsState, opts ...ResourceOption) (*FirewallOptions, error)
public static FirewallOptions Get(string name, Input<string> id, FirewallOptionsState? state, CustomResourceOptions? opts = null)
public static FirewallOptions get(String name, Output<String> id, FirewallOptionsState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Container
Id int - Container ID. Leave empty for cluster level aliases.
- Dhcp bool
- Enable DHCP.
- Enabled bool
- Enable or disable the firewall.
- Input
Policy string - The default input
policy (
ACCEPT
,DROP
,REJECT
). - Ipfilter bool
- Enable default IP filters. This is equivalent to
adding an empty
ipfilter-net<id>
ipset for every interface. Such ipsets implicitly contain sane default restrictions such as restricting IPv6 link local addresses to the one derived from the interface's MAC address. For containers the configured IP addresses will be implicitly added. - Log
Level stringIn - Log level for incoming
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - Log
Level stringOut - Log level for outgoing
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - Macfilter bool
- Enable/disable MAC address filter.
- Ndp bool
- Enable NDP (Neighbor Discovery Protocol).
- Node
Name string - Node name.
- Output
Policy string - The default output
policy (
ACCEPT
,DROP
,REJECT
). - Radv bool
- Enable Router Advertisement.
- Vm
Id int - VM ID. Leave empty for cluster level aliases.
- Container
Id int - Container ID. Leave empty for cluster level aliases.
- Dhcp bool
- Enable DHCP.
- Enabled bool
- Enable or disable the firewall.
- Input
Policy string - The default input
policy (
ACCEPT
,DROP
,REJECT
). - Ipfilter bool
- Enable default IP filters. This is equivalent to
adding an empty
ipfilter-net<id>
ipset for every interface. Such ipsets implicitly contain sane default restrictions such as restricting IPv6 link local addresses to the one derived from the interface's MAC address. For containers the configured IP addresses will be implicitly added. - Log
Level stringIn - Log level for incoming
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - Log
Level stringOut - Log level for outgoing
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - Macfilter bool
- Enable/disable MAC address filter.
- Ndp bool
- Enable NDP (Neighbor Discovery Protocol).
- Node
Name string - Node name.
- Output
Policy string - The default output
policy (
ACCEPT
,DROP
,REJECT
). - Radv bool
- Enable Router Advertisement.
- Vm
Id int - VM ID. Leave empty for cluster level aliases.
- container
Id Integer - Container ID. Leave empty for cluster level aliases.
- dhcp Boolean
- Enable DHCP.
- enabled Boolean
- Enable or disable the firewall.
- input
Policy String - The default input
policy (
ACCEPT
,DROP
,REJECT
). - ipfilter Boolean
- Enable default IP filters. This is equivalent to
adding an empty
ipfilter-net<id>
ipset for every interface. Such ipsets implicitly contain sane default restrictions such as restricting IPv6 link local addresses to the one derived from the interface's MAC address. For containers the configured IP addresses will be implicitly added. - log
Level StringIn - Log level for incoming
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - log
Level StringOut - Log level for outgoing
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - macfilter Boolean
- Enable/disable MAC address filter.
- ndp Boolean
- Enable NDP (Neighbor Discovery Protocol).
- node
Name String - Node name.
- output
Policy String - The default output
policy (
ACCEPT
,DROP
,REJECT
). - radv Boolean
- Enable Router Advertisement.
- vm
Id Integer - VM ID. Leave empty for cluster level aliases.
- container
Id number - Container ID. Leave empty for cluster level aliases.
- dhcp boolean
- Enable DHCP.
- enabled boolean
- Enable or disable the firewall.
- input
Policy string - The default input
policy (
ACCEPT
,DROP
,REJECT
). - ipfilter boolean
- Enable default IP filters. This is equivalent to
adding an empty
ipfilter-net<id>
ipset for every interface. Such ipsets implicitly contain sane default restrictions such as restricting IPv6 link local addresses to the one derived from the interface's MAC address. For containers the configured IP addresses will be implicitly added. - log
Level stringIn - Log level for incoming
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - log
Level stringOut - Log level for outgoing
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - macfilter boolean
- Enable/disable MAC address filter.
- ndp boolean
- Enable NDP (Neighbor Discovery Protocol).
- node
Name string - Node name.
- output
Policy string - The default output
policy (
ACCEPT
,DROP
,REJECT
). - radv boolean
- Enable Router Advertisement.
- vm
Id number - VM ID. Leave empty for cluster level aliases.
- container_
id int - Container ID. Leave empty for cluster level aliases.
- dhcp bool
- Enable DHCP.
- enabled bool
- Enable or disable the firewall.
- input_
policy str - The default input
policy (
ACCEPT
,DROP
,REJECT
). - ipfilter bool
- Enable default IP filters. This is equivalent to
adding an empty
ipfilter-net<id>
ipset for every interface. Such ipsets implicitly contain sane default restrictions such as restricting IPv6 link local addresses to the one derived from the interface's MAC address. For containers the configured IP addresses will be implicitly added. - log_
level_ strin - Log level for incoming
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - log_
level_ strout - Log level for outgoing
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - macfilter bool
- Enable/disable MAC address filter.
- ndp bool
- Enable NDP (Neighbor Discovery Protocol).
- node_
name str - Node name.
- output_
policy str - The default output
policy (
ACCEPT
,DROP
,REJECT
). - radv bool
- Enable Router Advertisement.
- vm_
id int - VM ID. Leave empty for cluster level aliases.
- container
Id Number - Container ID. Leave empty for cluster level aliases.
- dhcp Boolean
- Enable DHCP.
- enabled Boolean
- Enable or disable the firewall.
- input
Policy String - The default input
policy (
ACCEPT
,DROP
,REJECT
). - ipfilter Boolean
- Enable default IP filters. This is equivalent to
adding an empty
ipfilter-net<id>
ipset for every interface. Such ipsets implicitly contain sane default restrictions such as restricting IPv6 link local addresses to the one derived from the interface's MAC address. For containers the configured IP addresses will be implicitly added. - log
Level StringIn - Log level for incoming
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - log
Level StringOut - Log level for outgoing
packets (
emerg
,alert
,crit
,err
,warning
,notice
,info
,debug
,nolog
). - macfilter Boolean
- Enable/disable MAC address filter.
- ndp Boolean
- Enable NDP (Neighbor Discovery Protocol).
- node
Name String - Node name.
- output
Policy String - The default output
policy (
ACCEPT
,DROP
,REJECT
). - radv Boolean
- Enable Router Advertisement.
- vm
Id Number - VM ID. Leave empty for cluster level aliases.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
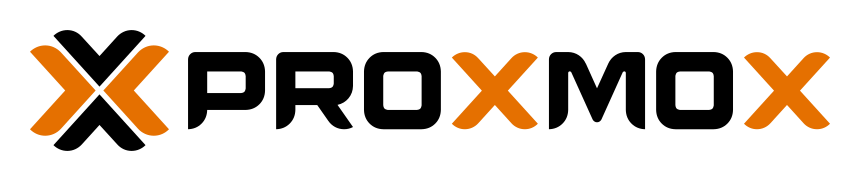