proxmoxve.HA.HAGroup
Explore with Pulumi AI
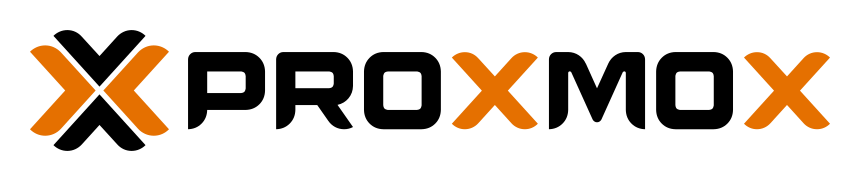
Manages a High Availability group in a Proxmox VE cluster.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@muhlba91/pulumi-proxmoxve";
const example = new proxmoxve.ha.HAGroup("example", {
group: "example",
comment: "This is a comment.",
nodes: {
node1: undefined,
node2: 2,
node3: 1,
},
restricted: true,
noFailback: false,
});
import pulumi
import pulumi_proxmoxve as proxmoxve
example = proxmoxve.ha.HAGroup("example",
group="example",
comment="This is a comment.",
nodes={
"node1": None,
"node2": 2,
"node3": 1,
},
restricted=True,
no_failback=False)
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/HA"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := HA.NewHAGroup(ctx, "example", &HA.HAGroupArgs{
Group: pulumi.String("example"),
Comment: pulumi.String("This is a comment."),
Nodes: pulumi.IntMap{
"node1": nil,
"node2": pulumi.Int(2),
"node3": pulumi.Int(1),
},
Restricted: pulumi.Bool(true),
NoFailback: pulumi.Bool(false),
})
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var example = new ProxmoxVE.HA.HAGroup("example", new()
{
Group = "example",
Comment = "This is a comment.",
Nodes =
{
{ "node1", null },
{ "node2", 2 },
{ "node3", 1 },
},
Restricted = true,
NoFailback = false,
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.HA.HAGroup;
import com.pulumi.proxmoxve.HA.HAGroupArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var example = new HAGroup("example", HAGroupArgs.builder()
.group("example")
.comment("This is a comment.")
.nodes(Map.ofEntries(
Map.entry("node1", null),
Map.entry("node2", 2),
Map.entry("node3", 1)
))
.restricted(true)
.noFailback(false)
.build());
}
}
resources:
example:
type: proxmoxve:HA:HAGroup
properties:
group: example
comment: This is a comment.
# Member nodes, with or without priority.
nodes:
node1: null
node2: 2
node3: 1
restricted: true
noFailback: false
Create HAGroup Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new HAGroup(name: string, args: HAGroupArgs, opts?: CustomResourceOptions);
@overload
def HAGroup(resource_name: str,
args: HAGroupArgs,
opts: Optional[ResourceOptions] = None)
@overload
def HAGroup(resource_name: str,
opts: Optional[ResourceOptions] = None,
group: Optional[str] = None,
nodes: Optional[Mapping[str, int]] = None,
comment: Optional[str] = None,
no_failback: Optional[bool] = None,
restricted: Optional[bool] = None)
func NewHAGroup(ctx *Context, name string, args HAGroupArgs, opts ...ResourceOption) (*HAGroup, error)
public HAGroup(string name, HAGroupArgs args, CustomResourceOptions? opts = null)
public HAGroup(String name, HAGroupArgs args)
public HAGroup(String name, HAGroupArgs args, CustomResourceOptions options)
type: proxmoxve:HA:HAGroup
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args HAGroupArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args HAGroupArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args HAGroupArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args HAGroupArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args HAGroupArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var hagroupResource = new ProxmoxVE.HA.HAGroup("hagroupResource", new()
{
Group = "string",
Nodes =
{
{ "string", 0 },
},
Comment = "string",
NoFailback = false,
Restricted = false,
});
example, err := HA.NewHAGroup(ctx, "hagroupResource", &HA.HAGroupArgs{
Group: pulumi.String("string"),
Nodes: pulumi.IntMap{
"string": pulumi.Int(0),
},
Comment: pulumi.String("string"),
NoFailback: pulumi.Bool(false),
Restricted: pulumi.Bool(false),
})
var hagroupResource = new HAGroup("hagroupResource", HAGroupArgs.builder()
.group("string")
.nodes(Map.of("string", 0))
.comment("string")
.noFailback(false)
.restricted(false)
.build());
hagroup_resource = proxmoxve.ha.HAGroup("hagroupResource",
group="string",
nodes={
"string": 0,
},
comment="string",
no_failback=False,
restricted=False)
const hagroupResource = new proxmoxve.ha.HAGroup("hagroupResource", {
group: "string",
nodes: {
string: 0,
},
comment: "string",
noFailback: false,
restricted: false,
});
type: proxmoxve:HA:HAGroup
properties:
comment: string
group: string
noFailback: false
nodes:
string: 0
restricted: false
HAGroup Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The HAGroup resource accepts the following input properties:
- Group string
- The identifier of the High Availability group to manage.
- Nodes Dictionary<string, int>
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - Comment string
- The comment associated with this group
- No
Failback bool - A flag that indicates that failing back to a higher priority node is disabled for this HA group. Defaults to
false
. - Restricted bool
- A flag that indicates that other nodes may not be used to run resources associated to this HA group. Defaults to
false
.
- Group string
- The identifier of the High Availability group to manage.
- Nodes map[string]int
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - Comment string
- The comment associated with this group
- No
Failback bool - A flag that indicates that failing back to a higher priority node is disabled for this HA group. Defaults to
false
. - Restricted bool
- A flag that indicates that other nodes may not be used to run resources associated to this HA group. Defaults to
false
.
- group String
- The identifier of the High Availability group to manage.
- nodes Map<String,Integer>
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - comment String
- The comment associated with this group
- no
Failback Boolean - A flag that indicates that failing back to a higher priority node is disabled for this HA group. Defaults to
false
. - restricted Boolean
- A flag that indicates that other nodes may not be used to run resources associated to this HA group. Defaults to
false
.
- group string
- The identifier of the High Availability group to manage.
- nodes {[key: string]: number}
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - comment string
- The comment associated with this group
- no
Failback boolean - A flag that indicates that failing back to a higher priority node is disabled for this HA group. Defaults to
false
. - restricted boolean
- A flag that indicates that other nodes may not be used to run resources associated to this HA group. Defaults to
false
.
- group str
- The identifier of the High Availability group to manage.
- nodes Mapping[str, int]
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - comment str
- The comment associated with this group
- no_
failback bool - A flag that indicates that failing back to a higher priority node is disabled for this HA group. Defaults to
false
. - restricted bool
- A flag that indicates that other nodes may not be used to run resources associated to this HA group. Defaults to
false
.
- group String
- The identifier of the High Availability group to manage.
- nodes Map<Number>
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - comment String
- The comment associated with this group
- no
Failback Boolean - A flag that indicates that failing back to a higher priority node is disabled for this HA group. Defaults to
false
. - restricted Boolean
- A flag that indicates that other nodes may not be used to run resources associated to this HA group. Defaults to
false
.
Outputs
All input properties are implicitly available as output properties. Additionally, the HAGroup resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing HAGroup Resource
Get an existing HAGroup resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: HAGroupState, opts?: CustomResourceOptions): HAGroup
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
comment: Optional[str] = None,
group: Optional[str] = None,
no_failback: Optional[bool] = None,
nodes: Optional[Mapping[str, int]] = None,
restricted: Optional[bool] = None) -> HAGroup
func GetHAGroup(ctx *Context, name string, id IDInput, state *HAGroupState, opts ...ResourceOption) (*HAGroup, error)
public static HAGroup Get(string name, Input<string> id, HAGroupState? state, CustomResourceOptions? opts = null)
public static HAGroup get(String name, Output<String> id, HAGroupState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Comment string
- The comment associated with this group
- Group string
- The identifier of the High Availability group to manage.
- No
Failback bool - A flag that indicates that failing back to a higher priority node is disabled for this HA group. Defaults to
false
. - Nodes Dictionary<string, int>
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - Restricted bool
- A flag that indicates that other nodes may not be used to run resources associated to this HA group. Defaults to
false
.
- Comment string
- The comment associated with this group
- Group string
- The identifier of the High Availability group to manage.
- No
Failback bool - A flag that indicates that failing back to a higher priority node is disabled for this HA group. Defaults to
false
. - Nodes map[string]int
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - Restricted bool
- A flag that indicates that other nodes may not be used to run resources associated to this HA group. Defaults to
false
.
- comment String
- The comment associated with this group
- group String
- The identifier of the High Availability group to manage.
- no
Failback Boolean - A flag that indicates that failing back to a higher priority node is disabled for this HA group. Defaults to
false
. - nodes Map<String,Integer>
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - restricted Boolean
- A flag that indicates that other nodes may not be used to run resources associated to this HA group. Defaults to
false
.
- comment string
- The comment associated with this group
- group string
- The identifier of the High Availability group to manage.
- no
Failback boolean - A flag that indicates that failing back to a higher priority node is disabled for this HA group. Defaults to
false
. - nodes {[key: string]: number}
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - restricted boolean
- A flag that indicates that other nodes may not be used to run resources associated to this HA group. Defaults to
false
.
- comment str
- The comment associated with this group
- group str
- The identifier of the High Availability group to manage.
- no_
failback bool - A flag that indicates that failing back to a higher priority node is disabled for this HA group. Defaults to
false
. - nodes Mapping[str, int]
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - restricted bool
- A flag that indicates that other nodes may not be used to run resources associated to this HA group. Defaults to
false
.
- comment String
- The comment associated with this group
- group String
- The identifier of the High Availability group to manage.
- no
Failback Boolean - A flag that indicates that failing back to a higher priority node is disabled for this HA group. Defaults to
false
. - nodes Map<Number>
- The member nodes for this group. They are provided as a map, where the keys are the node names and the values represent their priority: integers for known priorities or
null
for unset priorities. - restricted Boolean
- A flag that indicates that other nodes may not be used to run resources associated to this HA group. Defaults to
false
.
Import
#!/usr/bin/env sh
HA groups can be imported using their name, e.g.:
$ pulumi import proxmoxve:HA/hAGroup:HAGroup example example
To learn more about importing existing cloud resources, see Importing resources.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
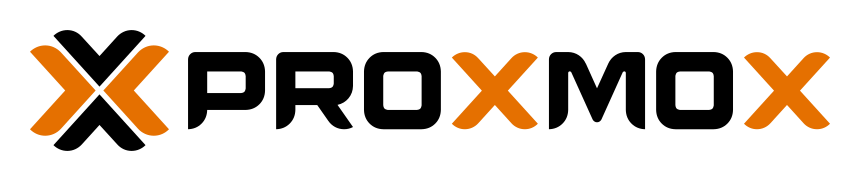