Proxmox Virtual Environment (Proxmox VE) v6.10.1 published on Friday, Jun 28, 2024 by Daniel Muehlbachler-Pietrzykowski
proxmoxve.HA.getHAResources
Explore with Pulumi AI
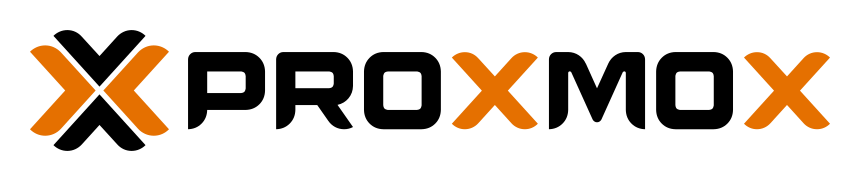
Proxmox Virtual Environment (Proxmox VE) v6.10.1 published on Friday, Jun 28, 2024 by Daniel Muehlbachler-Pietrzykowski
Retrieves the list of High Availability resources.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@pulumi/proxmoxve";
const exampleAll = proxmoxve.HA.getHAResources({});
const exampleVm = proxmoxve.HA.getHAResources({
type: "vm",
});
export const dataProxmoxVirtualEnvironmentHaresources = {
all: exampleAll.then(exampleAll => exampleAll.resourceIds),
vms: exampleVm.then(exampleVm => exampleVm.resourceIds),
};
import pulumi
import pulumi_proxmoxve as proxmoxve
example_all = proxmoxve.HA.get_ha_resources()
example_vm = proxmoxve.HA.get_ha_resources(type="vm")
pulumi.export("dataProxmoxVirtualEnvironmentHaresources", {
"all": example_all.resource_ids,
"vms": example_vm.resource_ids,
})
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/HA"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
exampleAll, err := HA.GetHAResources(ctx, nil, nil)
if err != nil {
return err
}
exampleVm, err := HA.GetHAResources(ctx, &ha.GetHAResourcesArgs{
Type: pulumi.StringRef("vm"),
}, nil)
if err != nil {
return err
}
ctx.Export("dataProxmoxVirtualEnvironmentHaresources", map[string]interface{}{
"all": exampleAll.ResourceIds,
"vms": exampleVm.ResourceIds,
})
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var exampleAll = ProxmoxVE.HA.GetHAResources.Invoke();
var exampleVm = ProxmoxVE.HA.GetHAResources.Invoke(new()
{
Type = "vm",
});
return new Dictionary<string, object?>
{
["dataProxmoxVirtualEnvironmentHaresources"] =
{
{ "all", exampleAll.Apply(getHAResourcesResult => getHAResourcesResult.ResourceIds) },
{ "vms", exampleVm.Apply(getHAResourcesResult => getHAResourcesResult.ResourceIds) },
},
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.HA.HAFunctions;
import com.pulumi.proxmoxve.HA.inputs.GetHAResourcesArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var exampleAll = HAFunctions.getHAResources();
final var exampleVm = HAFunctions.getHAResources(GetHAResourcesArgs.builder()
.type("vm")
.build());
ctx.export("dataProxmoxVirtualEnvironmentHaresources", %!v(PANIC=Format method: runtime error: invalid memory address or nil pointer dereference));
}
}
variables:
exampleAll:
fn::invoke:
Function: proxmoxve:HA:getHAResources
Arguments: {}
exampleVm:
fn::invoke:
Function: proxmoxve:HA:getHAResources
Arguments:
type: vm
outputs:
dataProxmoxVirtualEnvironmentHaresources:
all: ${exampleAll.resourceIds}
vms: ${exampleVm.resourceIds}
Using getHAResources
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getHAResources(args: GetHAResourcesArgs, opts?: InvokeOptions): Promise<GetHAResourcesResult>
function getHAResourcesOutput(args: GetHAResourcesOutputArgs, opts?: InvokeOptions): Output<GetHAResourcesResult>
def get_ha_resources(type: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetHAResourcesResult
def get_ha_resources_output(type: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetHAResourcesResult]
func GetHAResources(ctx *Context, args *GetHAResourcesArgs, opts ...InvokeOption) (*GetHAResourcesResult, error)
func GetHAResourcesOutput(ctx *Context, args *GetHAResourcesOutputArgs, opts ...InvokeOption) GetHAResourcesResultOutput
> Note: This function is named GetHAResources
in the Go SDK.
public static class GetHAResources
{
public static Task<GetHAResourcesResult> InvokeAsync(GetHAResourcesArgs args, InvokeOptions? opts = null)
public static Output<GetHAResourcesResult> Invoke(GetHAResourcesInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetHAResourcesResult> getHAResources(GetHAResourcesArgs args, InvokeOptions options)
// Output-based functions aren't available in Java yet
fn::invoke:
function: proxmoxve:HA/getHAResources:getHAResources
arguments:
# arguments dictionary
The following arguments are supported:
- Type string
- The type of High Availability resources to fetch (
vm
orct
). All resources will be fetched if this option is unset.
- Type string
- The type of High Availability resources to fetch (
vm
orct
). All resources will be fetched if this option is unset.
- type String
- The type of High Availability resources to fetch (
vm
orct
). All resources will be fetched if this option is unset.
- type string
- The type of High Availability resources to fetch (
vm
orct
). All resources will be fetched if this option is unset.
- type str
- The type of High Availability resources to fetch (
vm
orct
). All resources will be fetched if this option is unset.
- type String
- The type of High Availability resources to fetch (
vm
orct
). All resources will be fetched if this option is unset.
getHAResources Result
The following output properties are available:
- Id string
- The unique identifier of this resource.
- Resource
Ids List<string> - The identifiers of the High Availability resources.
- Type string
- The type of High Availability resources to fetch (
vm
orct
). All resources will be fetched if this option is unset.
- Id string
- The unique identifier of this resource.
- Resource
Ids []string - The identifiers of the High Availability resources.
- Type string
- The type of High Availability resources to fetch (
vm
orct
). All resources will be fetched if this option is unset.
- id String
- The unique identifier of this resource.
- resource
Ids List<String> - The identifiers of the High Availability resources.
- type String
- The type of High Availability resources to fetch (
vm
orct
). All resources will be fetched if this option is unset.
- id string
- The unique identifier of this resource.
- resource
Ids string[] - The identifiers of the High Availability resources.
- type string
- The type of High Availability resources to fetch (
vm
orct
). All resources will be fetched if this option is unset.
- id str
- The unique identifier of this resource.
- resource_
ids Sequence[str] - The identifiers of the High Availability resources.
- type str
- The type of High Availability resources to fetch (
vm
orct
). All resources will be fetched if this option is unset.
- id String
- The unique identifier of this resource.
- resource
Ids List<String> - The identifiers of the High Availability resources.
- type String
- The type of High Availability resources to fetch (
vm
orct
). All resources will be fetched if this option is unset.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
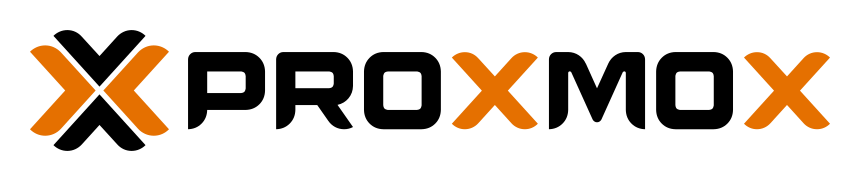
Proxmox Virtual Environment (Proxmox VE) v6.10.1 published on Friday, Jun 28, 2024 by Daniel Muehlbachler-Pietrzykowski