proxmoxve.Cluster.Options
Explore with Pulumi AI
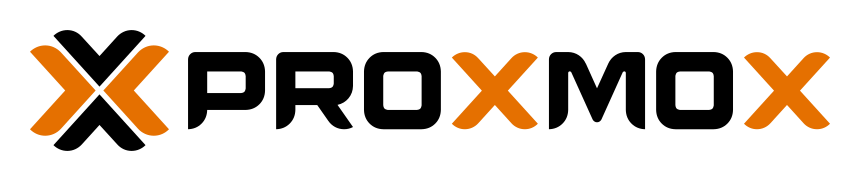
Manages Proxmox VE Cluster Datacenter options.
Example Usage
Coming soon!
Coming soon!
Coming soon!
Coming soon!
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Cluster.Options;
import com.pulumi.proxmoxve.Cluster.OptionsArgs;
import com.pulumi.proxmoxve.Cluster.inputs.OptionsNextIdArgs;
import com.pulumi.proxmoxve.Cluster.inputs.OptionsNotifyArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var options = new Options("options", OptionsArgs.builder()
.bandwidthLimitDefault(666666)
.bandwidthLimitMigration(555555)
.emailFrom("ged@gont.earthsea")
.keyboard("pl")
.language("en")
.maxWorkers(5)
.migrationCidr("10.0.0.0/8")
.migrationType("secure")
.nextId(OptionsNextIdArgs.builder()
.lower(100)
.upper(999999999)
.build())
.notify(OptionsNotifyArgs.builder()
.ha_fencing_mode("never")
.ha_fencing_target("default-matcher")
.package_replication("always")
.package_replication_target("default-matcher")
.package_updates("always")
.package_updates_target("default-matcher")
.build())
.build());
}
}
resources:
options:
type: proxmoxve:Cluster:Options
properties:
bandwidthLimitDefault: 666666
bandwidthLimitMigration: 555555
emailFrom: ged@gont.earthsea
keyboard: pl
language: en
maxWorkers: 5
migrationCidr: 10.0.0.0/8
migrationType: secure
nextId:
lower: 100
upper: 9.99999999e+08
notify:
ha_fencing_mode: never
ha_fencing_target: default-matcher
package_replication: always
package_replication_target: default-matcher
package_updates: always
package_updates_target: default-matcher
Create Options Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new Options(name: string, args?: OptionsArgs, opts?: CustomResourceOptions);
@overload
def Options(resource_name: str,
args: Optional[OptionsArgs] = None,
opts: Optional[ResourceOptions] = None)
@overload
def Options(resource_name: str,
opts: Optional[ResourceOptions] = None,
bandwidth_limit_clone: Optional[int] = None,
bandwidth_limit_default: Optional[int] = None,
bandwidth_limit_migration: Optional[int] = None,
bandwidth_limit_move: Optional[int] = None,
bandwidth_limit_restore: Optional[int] = None,
console: Optional[str] = None,
crs_ha: Optional[str] = None,
crs_ha_rebalance_on_start: Optional[bool] = None,
description: Optional[str] = None,
email_from: Optional[str] = None,
ha_shutdown_policy: Optional[str] = None,
http_proxy: Optional[str] = None,
keyboard: Optional[str] = None,
language: Optional[str] = None,
mac_prefix: Optional[str] = None,
max_workers: Optional[int] = None,
migration_cidr: Optional[str] = None,
migration_type: Optional[str] = None,
next_id: Optional[_cluster.OptionsNextIdArgs] = None,
notify: Optional[_cluster.OptionsNotifyArgs] = None)
func NewOptions(ctx *Context, name string, args *OptionsArgs, opts ...ResourceOption) (*Options, error)
public Options(string name, OptionsArgs? args = null, CustomResourceOptions? opts = null)
public Options(String name, OptionsArgs args)
public Options(String name, OptionsArgs args, CustomResourceOptions options)
type: proxmoxve:Cluster:Options
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args OptionsArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args OptionsArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args OptionsArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args OptionsArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args OptionsArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var optionsResource = new ProxmoxVE.Cluster.Options("optionsResource", new()
{
BandwidthLimitClone = 0,
BandwidthLimitDefault = 0,
BandwidthLimitMigration = 0,
BandwidthLimitMove = 0,
BandwidthLimitRestore = 0,
Console = "string",
CrsHa = "string",
CrsHaRebalanceOnStart = false,
Description = "string",
EmailFrom = "string",
HaShutdownPolicy = "string",
HttpProxy = "string",
Keyboard = "string",
Language = "string",
MacPrefix = "string",
MaxWorkers = 0,
MigrationCidr = "string",
MigrationType = "string",
NextId = new ProxmoxVE.Cluster.Inputs.OptionsNextIdArgs
{
Lower = 0,
Upper = 0,
},
Notify = new ProxmoxVE.Cluster.Inputs.OptionsNotifyArgs
{
HaFencingMode = "string",
HaFencingTarget = "string",
PackageUpdates = "string",
PackageUpdatesTarget = "string",
Replication = "string",
ReplicationTarget = "string",
},
});
example, err := Cluster.NewOptions(ctx, "optionsResource", &Cluster.OptionsArgs{
BandwidthLimitClone: pulumi.Int(0),
BandwidthLimitDefault: pulumi.Int(0),
BandwidthLimitMigration: pulumi.Int(0),
BandwidthLimitMove: pulumi.Int(0),
BandwidthLimitRestore: pulumi.Int(0),
Console: pulumi.String("string"),
CrsHa: pulumi.String("string"),
CrsHaRebalanceOnStart: pulumi.Bool(false),
Description: pulumi.String("string"),
EmailFrom: pulumi.String("string"),
HaShutdownPolicy: pulumi.String("string"),
HttpProxy: pulumi.String("string"),
Keyboard: pulumi.String("string"),
Language: pulumi.String("string"),
MacPrefix: pulumi.String("string"),
MaxWorkers: pulumi.Int(0),
MigrationCidr: pulumi.String("string"),
MigrationType: pulumi.String("string"),
NextId: &cluster.OptionsNextIdArgs{
Lower: pulumi.Int(0),
Upper: pulumi.Int(0),
},
Notify: &cluster.OptionsNotifyArgs{
HaFencingMode: pulumi.String("string"),
HaFencingTarget: pulumi.String("string"),
PackageUpdates: pulumi.String("string"),
PackageUpdatesTarget: pulumi.String("string"),
Replication: pulumi.String("string"),
ReplicationTarget: pulumi.String("string"),
},
})
var optionsResource = new Options("optionsResource", OptionsArgs.builder()
.bandwidthLimitClone(0)
.bandwidthLimitDefault(0)
.bandwidthLimitMigration(0)
.bandwidthLimitMove(0)
.bandwidthLimitRestore(0)
.console("string")
.crsHa("string")
.crsHaRebalanceOnStart(false)
.description("string")
.emailFrom("string")
.haShutdownPolicy("string")
.httpProxy("string")
.keyboard("string")
.language("string")
.macPrefix("string")
.maxWorkers(0)
.migrationCidr("string")
.migrationType("string")
.nextId(OptionsNextIdArgs.builder()
.lower(0)
.upper(0)
.build())
.notify(OptionsNotifyArgs.builder()
.haFencingMode("string")
.haFencingTarget("string")
.packageUpdates("string")
.packageUpdatesTarget("string")
.replication("string")
.replicationTarget("string")
.build())
.build());
options_resource = proxmoxve.cluster.Options("optionsResource",
bandwidth_limit_clone=0,
bandwidth_limit_default=0,
bandwidth_limit_migration=0,
bandwidth_limit_move=0,
bandwidth_limit_restore=0,
console="string",
crs_ha="string",
crs_ha_rebalance_on_start=False,
description="string",
email_from="string",
ha_shutdown_policy="string",
http_proxy="string",
keyboard="string",
language="string",
mac_prefix="string",
max_workers=0,
migration_cidr="string",
migration_type="string",
next_id=proxmoxve.cluster.OptionsNextIdArgs(
lower=0,
upper=0,
),
notify=proxmoxve.cluster.OptionsNotifyArgs(
ha_fencing_mode="string",
ha_fencing_target="string",
package_updates="string",
package_updates_target="string",
replication="string",
replication_target="string",
))
const optionsResource = new proxmoxve.cluster.Options("optionsResource", {
bandwidthLimitClone: 0,
bandwidthLimitDefault: 0,
bandwidthLimitMigration: 0,
bandwidthLimitMove: 0,
bandwidthLimitRestore: 0,
console: "string",
crsHa: "string",
crsHaRebalanceOnStart: false,
description: "string",
emailFrom: "string",
haShutdownPolicy: "string",
httpProxy: "string",
keyboard: "string",
language: "string",
macPrefix: "string",
maxWorkers: 0,
migrationCidr: "string",
migrationType: "string",
nextId: {
lower: 0,
upper: 0,
},
notify: {
haFencingMode: "string",
haFencingTarget: "string",
packageUpdates: "string",
packageUpdatesTarget: "string",
replication: "string",
replicationTarget: "string",
},
});
type: proxmoxve:Cluster:Options
properties:
bandwidthLimitClone: 0
bandwidthLimitDefault: 0
bandwidthLimitMigration: 0
bandwidthLimitMove: 0
bandwidthLimitRestore: 0
console: string
crsHa: string
crsHaRebalanceOnStart: false
description: string
emailFrom: string
haShutdownPolicy: string
httpProxy: string
keyboard: string
language: string
macPrefix: string
maxWorkers: 0
migrationCidr: string
migrationType: string
nextId:
lower: 0
upper: 0
notify:
haFencingMode: string
haFencingTarget: string
packageUpdates: string
packageUpdatesTarget: string
replication: string
replicationTarget: string
Options Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The Options resource accepts the following input properties:
- Bandwidth
Limit intClone - Clone I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intDefault - Default I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intMigration - Migration I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intMove - Move I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intRestore - Restore I/O bandwidth limit in KiB/s.
- Console string
- Select the default Console viewer. Must be
applet
|vv
|html5
|xtermjs
. You can either use the builtin java applet (VNC; deprecated and maps to html5), an external virt-viewer compatible application (SPICE), an HTML5 based vnc viewer (noVNC), or an HTML5 based console client (xtermjs). If the selected viewer is not available (e.g. SPICE not activated for the VM), the fallback is noVNC. - Crs
Ha string - Cluster resource scheduling setting for HA. Must be
static
|basic
(default isbasic
). - bool
- Cluster resource scheduling setting for HA rebalance on start.
- Description string
- Datacenter description. Shown in the web-interface datacenter notes panel. This is saved as comment inside the configuration file.
- Email
From string - email address to send notification from (default is root@$hostname).
- Ha
Shutdown stringPolicy - Cluster wide HA shutdown policy (). Must be
freeze
|failover
|migrate
|conditional
(default isconditional
). - Http
Proxy string - Specify external http proxy which is used for downloads (example:
http://username:password@host:port/
). - Keyboard string
- Default keyboard layout for vnc server. Must be
de
|de-ch
|da
|en-gb
|en-us
|es
|fi
|fr
|fr-be
|fr-ca
|fr-ch
|hu
|is
|it
|ja
|lt
|mk
|nl
|no
|pl
|pt
|pt-br
|sv
|sl
|tr
. - Language string
- Default GUI language. Must be
ca
|da
|de
|en
|es
|eu
|fa
|fr
|he
|it
|ja
|nb
|nn
|pl
|pt_BR
|ru
|sl
|sv
|tr
|zh_CN
|zh_TW
. - Mac
Prefix string - Prefix for autogenerated MAC addresses.
- Max
Workers int - Defines how many workers (per node) are maximal started on actions like 'stopall VMs' or task from the ha-manager.
- Migration
Cidr string - Cluster wide migration network CIDR.
- Migration
Type string - Cluster wide migration type. Must be
secure
|unsecure
(default issecure
). - Next
Id Pulumi.Proxmox VE. Cluster. Inputs. Options Next Id - The ranges for the next free VM ID auto-selection pool.
- Notify
Pulumi.
Proxmox VE. Cluster. Inputs. Options Notify - Cluster-wide notification settings.
- Bandwidth
Limit intClone - Clone I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intDefault - Default I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intMigration - Migration I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intMove - Move I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intRestore - Restore I/O bandwidth limit in KiB/s.
- Console string
- Select the default Console viewer. Must be
applet
|vv
|html5
|xtermjs
. You can either use the builtin java applet (VNC; deprecated and maps to html5), an external virt-viewer compatible application (SPICE), an HTML5 based vnc viewer (noVNC), or an HTML5 based console client (xtermjs). If the selected viewer is not available (e.g. SPICE not activated for the VM), the fallback is noVNC. - Crs
Ha string - Cluster resource scheduling setting for HA. Must be
static
|basic
(default isbasic
). - bool
- Cluster resource scheduling setting for HA rebalance on start.
- Description string
- Datacenter description. Shown in the web-interface datacenter notes panel. This is saved as comment inside the configuration file.
- Email
From string - email address to send notification from (default is root@$hostname).
- Ha
Shutdown stringPolicy - Cluster wide HA shutdown policy (). Must be
freeze
|failover
|migrate
|conditional
(default isconditional
). - Http
Proxy string - Specify external http proxy which is used for downloads (example:
http://username:password@host:port/
). - Keyboard string
- Default keyboard layout for vnc server. Must be
de
|de-ch
|da
|en-gb
|en-us
|es
|fi
|fr
|fr-be
|fr-ca
|fr-ch
|hu
|is
|it
|ja
|lt
|mk
|nl
|no
|pl
|pt
|pt-br
|sv
|sl
|tr
. - Language string
- Default GUI language. Must be
ca
|da
|de
|en
|es
|eu
|fa
|fr
|he
|it
|ja
|nb
|nn
|pl
|pt_BR
|ru
|sl
|sv
|tr
|zh_CN
|zh_TW
. - Mac
Prefix string - Prefix for autogenerated MAC addresses.
- Max
Workers int - Defines how many workers (per node) are maximal started on actions like 'stopall VMs' or task from the ha-manager.
- Migration
Cidr string - Cluster wide migration network CIDR.
- Migration
Type string - Cluster wide migration type. Must be
secure
|unsecure
(default issecure
). - Next
Id OptionsNext Id Args - The ranges for the next free VM ID auto-selection pool.
- Notify
Options
Notify Args - Cluster-wide notification settings.
- bandwidth
Limit IntegerClone - Clone I/O bandwidth limit in KiB/s.
- bandwidth
Limit IntegerDefault - Default I/O bandwidth limit in KiB/s.
- bandwidth
Limit IntegerMigration - Migration I/O bandwidth limit in KiB/s.
- bandwidth
Limit IntegerMove - Move I/O bandwidth limit in KiB/s.
- bandwidth
Limit IntegerRestore - Restore I/O bandwidth limit in KiB/s.
- console String
- Select the default Console viewer. Must be
applet
|vv
|html5
|xtermjs
. You can either use the builtin java applet (VNC; deprecated and maps to html5), an external virt-viewer compatible application (SPICE), an HTML5 based vnc viewer (noVNC), or an HTML5 based console client (xtermjs). If the selected viewer is not available (e.g. SPICE not activated for the VM), the fallback is noVNC. - crs
Ha String - Cluster resource scheduling setting for HA. Must be
static
|basic
(default isbasic
). - Boolean
- Cluster resource scheduling setting for HA rebalance on start.
- description String
- Datacenter description. Shown in the web-interface datacenter notes panel. This is saved as comment inside the configuration file.
- email
From String - email address to send notification from (default is root@$hostname).
- ha
Shutdown StringPolicy - Cluster wide HA shutdown policy (). Must be
freeze
|failover
|migrate
|conditional
(default isconditional
). - http
Proxy String - Specify external http proxy which is used for downloads (example:
http://username:password@host:port/
). - keyboard String
- Default keyboard layout for vnc server. Must be
de
|de-ch
|da
|en-gb
|en-us
|es
|fi
|fr
|fr-be
|fr-ca
|fr-ch
|hu
|is
|it
|ja
|lt
|mk
|nl
|no
|pl
|pt
|pt-br
|sv
|sl
|tr
. - language String
- Default GUI language. Must be
ca
|da
|de
|en
|es
|eu
|fa
|fr
|he
|it
|ja
|nb
|nn
|pl
|pt_BR
|ru
|sl
|sv
|tr
|zh_CN
|zh_TW
. - mac
Prefix String - Prefix for autogenerated MAC addresses.
- max
Workers Integer - Defines how many workers (per node) are maximal started on actions like 'stopall VMs' or task from the ha-manager.
- migration
Cidr String - Cluster wide migration network CIDR.
- migration
Type String - Cluster wide migration type. Must be
secure
|unsecure
(default issecure
). - next
Id OptionsNext Id - The ranges for the next free VM ID auto-selection pool.
- notify_
Options
Notify - Cluster-wide notification settings.
- bandwidth
Limit numberClone - Clone I/O bandwidth limit in KiB/s.
- bandwidth
Limit numberDefault - Default I/O bandwidth limit in KiB/s.
- bandwidth
Limit numberMigration - Migration I/O bandwidth limit in KiB/s.
- bandwidth
Limit numberMove - Move I/O bandwidth limit in KiB/s.
- bandwidth
Limit numberRestore - Restore I/O bandwidth limit in KiB/s.
- console string
- Select the default Console viewer. Must be
applet
|vv
|html5
|xtermjs
. You can either use the builtin java applet (VNC; deprecated and maps to html5), an external virt-viewer compatible application (SPICE), an HTML5 based vnc viewer (noVNC), or an HTML5 based console client (xtermjs). If the selected viewer is not available (e.g. SPICE not activated for the VM), the fallback is noVNC. - crs
Ha string - Cluster resource scheduling setting for HA. Must be
static
|basic
(default isbasic
). - boolean
- Cluster resource scheduling setting for HA rebalance on start.
- description string
- Datacenter description. Shown in the web-interface datacenter notes panel. This is saved as comment inside the configuration file.
- email
From string - email address to send notification from (default is root@$hostname).
- ha
Shutdown stringPolicy - Cluster wide HA shutdown policy (). Must be
freeze
|failover
|migrate
|conditional
(default isconditional
). - http
Proxy string - Specify external http proxy which is used for downloads (example:
http://username:password@host:port/
). - keyboard string
- Default keyboard layout for vnc server. Must be
de
|de-ch
|da
|en-gb
|en-us
|es
|fi
|fr
|fr-be
|fr-ca
|fr-ch
|hu
|is
|it
|ja
|lt
|mk
|nl
|no
|pl
|pt
|pt-br
|sv
|sl
|tr
. - language string
- Default GUI language. Must be
ca
|da
|de
|en
|es
|eu
|fa
|fr
|he
|it
|ja
|nb
|nn
|pl
|pt_BR
|ru
|sl
|sv
|tr
|zh_CN
|zh_TW
. - mac
Prefix string - Prefix for autogenerated MAC addresses.
- max
Workers number - Defines how many workers (per node) are maximal started on actions like 'stopall VMs' or task from the ha-manager.
- migration
Cidr string - Cluster wide migration network CIDR.
- migration
Type string - Cluster wide migration type. Must be
secure
|unsecure
(default issecure
). - next
Id OptionsNext Id - The ranges for the next free VM ID auto-selection pool.
- notify
Options
Notify - Cluster-wide notification settings.
- bandwidth_
limit_ intclone - Clone I/O bandwidth limit in KiB/s.
- bandwidth_
limit_ intdefault - Default I/O bandwidth limit in KiB/s.
- bandwidth_
limit_ intmigration - Migration I/O bandwidth limit in KiB/s.
- bandwidth_
limit_ intmove - Move I/O bandwidth limit in KiB/s.
- bandwidth_
limit_ intrestore - Restore I/O bandwidth limit in KiB/s.
- console str
- Select the default Console viewer. Must be
applet
|vv
|html5
|xtermjs
. You can either use the builtin java applet (VNC; deprecated and maps to html5), an external virt-viewer compatible application (SPICE), an HTML5 based vnc viewer (noVNC), or an HTML5 based console client (xtermjs). If the selected viewer is not available (e.g. SPICE not activated for the VM), the fallback is noVNC. - crs_
ha str - Cluster resource scheduling setting for HA. Must be
static
|basic
(default isbasic
). - crs_
ha_ boolrebalance_ on_ start - Cluster resource scheduling setting for HA rebalance on start.
- description str
- Datacenter description. Shown in the web-interface datacenter notes panel. This is saved as comment inside the configuration file.
- email_
from str - email address to send notification from (default is root@$hostname).
- ha_
shutdown_ strpolicy - Cluster wide HA shutdown policy (). Must be
freeze
|failover
|migrate
|conditional
(default isconditional
). - http_
proxy str - Specify external http proxy which is used for downloads (example:
http://username:password@host:port/
). - keyboard str
- Default keyboard layout for vnc server. Must be
de
|de-ch
|da
|en-gb
|en-us
|es
|fi
|fr
|fr-be
|fr-ca
|fr-ch
|hu
|is
|it
|ja
|lt
|mk
|nl
|no
|pl
|pt
|pt-br
|sv
|sl
|tr
. - language str
- Default GUI language. Must be
ca
|da
|de
|en
|es
|eu
|fa
|fr
|he
|it
|ja
|nb
|nn
|pl
|pt_BR
|ru
|sl
|sv
|tr
|zh_CN
|zh_TW
. - mac_
prefix str - Prefix for autogenerated MAC addresses.
- max_
workers int - Defines how many workers (per node) are maximal started on actions like 'stopall VMs' or task from the ha-manager.
- migration_
cidr str - Cluster wide migration network CIDR.
- migration_
type str - Cluster wide migration type. Must be
secure
|unsecure
(default issecure
). - next_
id cluster.Options Next Id Args - The ranges for the next free VM ID auto-selection pool.
- notify
cluster.
Options Notify Args - Cluster-wide notification settings.
- bandwidth
Limit NumberClone - Clone I/O bandwidth limit in KiB/s.
- bandwidth
Limit NumberDefault - Default I/O bandwidth limit in KiB/s.
- bandwidth
Limit NumberMigration - Migration I/O bandwidth limit in KiB/s.
- bandwidth
Limit NumberMove - Move I/O bandwidth limit in KiB/s.
- bandwidth
Limit NumberRestore - Restore I/O bandwidth limit in KiB/s.
- console String
- Select the default Console viewer. Must be
applet
|vv
|html5
|xtermjs
. You can either use the builtin java applet (VNC; deprecated and maps to html5), an external virt-viewer compatible application (SPICE), an HTML5 based vnc viewer (noVNC), or an HTML5 based console client (xtermjs). If the selected viewer is not available (e.g. SPICE not activated for the VM), the fallback is noVNC. - crs
Ha String - Cluster resource scheduling setting for HA. Must be
static
|basic
(default isbasic
). - Boolean
- Cluster resource scheduling setting for HA rebalance on start.
- description String
- Datacenter description. Shown in the web-interface datacenter notes panel. This is saved as comment inside the configuration file.
- email
From String - email address to send notification from (default is root@$hostname).
- ha
Shutdown StringPolicy - Cluster wide HA shutdown policy (). Must be
freeze
|failover
|migrate
|conditional
(default isconditional
). - http
Proxy String - Specify external http proxy which is used for downloads (example:
http://username:password@host:port/
). - keyboard String
- Default keyboard layout for vnc server. Must be
de
|de-ch
|da
|en-gb
|en-us
|es
|fi
|fr
|fr-be
|fr-ca
|fr-ch
|hu
|is
|it
|ja
|lt
|mk
|nl
|no
|pl
|pt
|pt-br
|sv
|sl
|tr
. - language String
- Default GUI language. Must be
ca
|da
|de
|en
|es
|eu
|fa
|fr
|he
|it
|ja
|nb
|nn
|pl
|pt_BR
|ru
|sl
|sv
|tr
|zh_CN
|zh_TW
. - mac
Prefix String - Prefix for autogenerated MAC addresses.
- max
Workers Number - Defines how many workers (per node) are maximal started on actions like 'stopall VMs' or task from the ha-manager.
- migration
Cidr String - Cluster wide migration network CIDR.
- migration
Type String - Cluster wide migration type. Must be
secure
|unsecure
(default issecure
). - next
Id Property Map - The ranges for the next free VM ID auto-selection pool.
- notify Property Map
- Cluster-wide notification settings.
Outputs
All input properties are implicitly available as output properties. Additionally, the Options resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing Options Resource
Get an existing Options resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: OptionsState, opts?: CustomResourceOptions): Options
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
bandwidth_limit_clone: Optional[int] = None,
bandwidth_limit_default: Optional[int] = None,
bandwidth_limit_migration: Optional[int] = None,
bandwidth_limit_move: Optional[int] = None,
bandwidth_limit_restore: Optional[int] = None,
console: Optional[str] = None,
crs_ha: Optional[str] = None,
crs_ha_rebalance_on_start: Optional[bool] = None,
description: Optional[str] = None,
email_from: Optional[str] = None,
ha_shutdown_policy: Optional[str] = None,
http_proxy: Optional[str] = None,
keyboard: Optional[str] = None,
language: Optional[str] = None,
mac_prefix: Optional[str] = None,
max_workers: Optional[int] = None,
migration_cidr: Optional[str] = None,
migration_type: Optional[str] = None,
next_id: Optional[_cluster.OptionsNextIdArgs] = None,
notify: Optional[_cluster.OptionsNotifyArgs] = None) -> Options
func GetOptions(ctx *Context, name string, id IDInput, state *OptionsState, opts ...ResourceOption) (*Options, error)
public static Options Get(string name, Input<string> id, OptionsState? state, CustomResourceOptions? opts = null)
public static Options get(String name, Output<String> id, OptionsState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Bandwidth
Limit intClone - Clone I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intDefault - Default I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intMigration - Migration I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intMove - Move I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intRestore - Restore I/O bandwidth limit in KiB/s.
- Console string
- Select the default Console viewer. Must be
applet
|vv
|html5
|xtermjs
. You can either use the builtin java applet (VNC; deprecated and maps to html5), an external virt-viewer compatible application (SPICE), an HTML5 based vnc viewer (noVNC), or an HTML5 based console client (xtermjs). If the selected viewer is not available (e.g. SPICE not activated for the VM), the fallback is noVNC. - Crs
Ha string - Cluster resource scheduling setting for HA. Must be
static
|basic
(default isbasic
). - bool
- Cluster resource scheduling setting for HA rebalance on start.
- Description string
- Datacenter description. Shown in the web-interface datacenter notes panel. This is saved as comment inside the configuration file.
- Email
From string - email address to send notification from (default is root@$hostname).
- Ha
Shutdown stringPolicy - Cluster wide HA shutdown policy (). Must be
freeze
|failover
|migrate
|conditional
(default isconditional
). - Http
Proxy string - Specify external http proxy which is used for downloads (example:
http://username:password@host:port/
). - Keyboard string
- Default keyboard layout for vnc server. Must be
de
|de-ch
|da
|en-gb
|en-us
|es
|fi
|fr
|fr-be
|fr-ca
|fr-ch
|hu
|is
|it
|ja
|lt
|mk
|nl
|no
|pl
|pt
|pt-br
|sv
|sl
|tr
. - Language string
- Default GUI language. Must be
ca
|da
|de
|en
|es
|eu
|fa
|fr
|he
|it
|ja
|nb
|nn
|pl
|pt_BR
|ru
|sl
|sv
|tr
|zh_CN
|zh_TW
. - Mac
Prefix string - Prefix for autogenerated MAC addresses.
- Max
Workers int - Defines how many workers (per node) are maximal started on actions like 'stopall VMs' or task from the ha-manager.
- Migration
Cidr string - Cluster wide migration network CIDR.
- Migration
Type string - Cluster wide migration type. Must be
secure
|unsecure
(default issecure
). - Next
Id Pulumi.Proxmox VE. Cluster. Inputs. Options Next Id - The ranges for the next free VM ID auto-selection pool.
- Notify
Pulumi.
Proxmox VE. Cluster. Inputs. Options Notify - Cluster-wide notification settings.
- Bandwidth
Limit intClone - Clone I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intDefault - Default I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intMigration - Migration I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intMove - Move I/O bandwidth limit in KiB/s.
- Bandwidth
Limit intRestore - Restore I/O bandwidth limit in KiB/s.
- Console string
- Select the default Console viewer. Must be
applet
|vv
|html5
|xtermjs
. You can either use the builtin java applet (VNC; deprecated and maps to html5), an external virt-viewer compatible application (SPICE), an HTML5 based vnc viewer (noVNC), or an HTML5 based console client (xtermjs). If the selected viewer is not available (e.g. SPICE not activated for the VM), the fallback is noVNC. - Crs
Ha string - Cluster resource scheduling setting for HA. Must be
static
|basic
(default isbasic
). - bool
- Cluster resource scheduling setting for HA rebalance on start.
- Description string
- Datacenter description. Shown in the web-interface datacenter notes panel. This is saved as comment inside the configuration file.
- Email
From string - email address to send notification from (default is root@$hostname).
- Ha
Shutdown stringPolicy - Cluster wide HA shutdown policy (). Must be
freeze
|failover
|migrate
|conditional
(default isconditional
). - Http
Proxy string - Specify external http proxy which is used for downloads (example:
http://username:password@host:port/
). - Keyboard string
- Default keyboard layout for vnc server. Must be
de
|de-ch
|da
|en-gb
|en-us
|es
|fi
|fr
|fr-be
|fr-ca
|fr-ch
|hu
|is
|it
|ja
|lt
|mk
|nl
|no
|pl
|pt
|pt-br
|sv
|sl
|tr
. - Language string
- Default GUI language. Must be
ca
|da
|de
|en
|es
|eu
|fa
|fr
|he
|it
|ja
|nb
|nn
|pl
|pt_BR
|ru
|sl
|sv
|tr
|zh_CN
|zh_TW
. - Mac
Prefix string - Prefix for autogenerated MAC addresses.
- Max
Workers int - Defines how many workers (per node) are maximal started on actions like 'stopall VMs' or task from the ha-manager.
- Migration
Cidr string - Cluster wide migration network CIDR.
- Migration
Type string - Cluster wide migration type. Must be
secure
|unsecure
(default issecure
). - Next
Id OptionsNext Id Args - The ranges for the next free VM ID auto-selection pool.
- Notify
Options
Notify Args - Cluster-wide notification settings.
- bandwidth
Limit IntegerClone - Clone I/O bandwidth limit in KiB/s.
- bandwidth
Limit IntegerDefault - Default I/O bandwidth limit in KiB/s.
- bandwidth
Limit IntegerMigration - Migration I/O bandwidth limit in KiB/s.
- bandwidth
Limit IntegerMove - Move I/O bandwidth limit in KiB/s.
- bandwidth
Limit IntegerRestore - Restore I/O bandwidth limit in KiB/s.
- console String
- Select the default Console viewer. Must be
applet
|vv
|html5
|xtermjs
. You can either use the builtin java applet (VNC; deprecated and maps to html5), an external virt-viewer compatible application (SPICE), an HTML5 based vnc viewer (noVNC), or an HTML5 based console client (xtermjs). If the selected viewer is not available (e.g. SPICE not activated for the VM), the fallback is noVNC. - crs
Ha String - Cluster resource scheduling setting for HA. Must be
static
|basic
(default isbasic
). - Boolean
- Cluster resource scheduling setting for HA rebalance on start.
- description String
- Datacenter description. Shown in the web-interface datacenter notes panel. This is saved as comment inside the configuration file.
- email
From String - email address to send notification from (default is root@$hostname).
- ha
Shutdown StringPolicy - Cluster wide HA shutdown policy (). Must be
freeze
|failover
|migrate
|conditional
(default isconditional
). - http
Proxy String - Specify external http proxy which is used for downloads (example:
http://username:password@host:port/
). - keyboard String
- Default keyboard layout for vnc server. Must be
de
|de-ch
|da
|en-gb
|en-us
|es
|fi
|fr
|fr-be
|fr-ca
|fr-ch
|hu
|is
|it
|ja
|lt
|mk
|nl
|no
|pl
|pt
|pt-br
|sv
|sl
|tr
. - language String
- Default GUI language. Must be
ca
|da
|de
|en
|es
|eu
|fa
|fr
|he
|it
|ja
|nb
|nn
|pl
|pt_BR
|ru
|sl
|sv
|tr
|zh_CN
|zh_TW
. - mac
Prefix String - Prefix for autogenerated MAC addresses.
- max
Workers Integer - Defines how many workers (per node) are maximal started on actions like 'stopall VMs' or task from the ha-manager.
- migration
Cidr String - Cluster wide migration network CIDR.
- migration
Type String - Cluster wide migration type. Must be
secure
|unsecure
(default issecure
). - next
Id OptionsNext Id - The ranges for the next free VM ID auto-selection pool.
- notify_
Options
Notify - Cluster-wide notification settings.
- bandwidth
Limit numberClone - Clone I/O bandwidth limit in KiB/s.
- bandwidth
Limit numberDefault - Default I/O bandwidth limit in KiB/s.
- bandwidth
Limit numberMigration - Migration I/O bandwidth limit in KiB/s.
- bandwidth
Limit numberMove - Move I/O bandwidth limit in KiB/s.
- bandwidth
Limit numberRestore - Restore I/O bandwidth limit in KiB/s.
- console string
- Select the default Console viewer. Must be
applet
|vv
|html5
|xtermjs
. You can either use the builtin java applet (VNC; deprecated and maps to html5), an external virt-viewer compatible application (SPICE), an HTML5 based vnc viewer (noVNC), or an HTML5 based console client (xtermjs). If the selected viewer is not available (e.g. SPICE not activated for the VM), the fallback is noVNC. - crs
Ha string - Cluster resource scheduling setting for HA. Must be
static
|basic
(default isbasic
). - boolean
- Cluster resource scheduling setting for HA rebalance on start.
- description string
- Datacenter description. Shown in the web-interface datacenter notes panel. This is saved as comment inside the configuration file.
- email
From string - email address to send notification from (default is root@$hostname).
- ha
Shutdown stringPolicy - Cluster wide HA shutdown policy (). Must be
freeze
|failover
|migrate
|conditional
(default isconditional
). - http
Proxy string - Specify external http proxy which is used for downloads (example:
http://username:password@host:port/
). - keyboard string
- Default keyboard layout for vnc server. Must be
de
|de-ch
|da
|en-gb
|en-us
|es
|fi
|fr
|fr-be
|fr-ca
|fr-ch
|hu
|is
|it
|ja
|lt
|mk
|nl
|no
|pl
|pt
|pt-br
|sv
|sl
|tr
. - language string
- Default GUI language. Must be
ca
|da
|de
|en
|es
|eu
|fa
|fr
|he
|it
|ja
|nb
|nn
|pl
|pt_BR
|ru
|sl
|sv
|tr
|zh_CN
|zh_TW
. - mac
Prefix string - Prefix for autogenerated MAC addresses.
- max
Workers number - Defines how many workers (per node) are maximal started on actions like 'stopall VMs' or task from the ha-manager.
- migration
Cidr string - Cluster wide migration network CIDR.
- migration
Type string - Cluster wide migration type. Must be
secure
|unsecure
(default issecure
). - next
Id OptionsNext Id - The ranges for the next free VM ID auto-selection pool.
- notify
Options
Notify - Cluster-wide notification settings.
- bandwidth_
limit_ intclone - Clone I/O bandwidth limit in KiB/s.
- bandwidth_
limit_ intdefault - Default I/O bandwidth limit in KiB/s.
- bandwidth_
limit_ intmigration - Migration I/O bandwidth limit in KiB/s.
- bandwidth_
limit_ intmove - Move I/O bandwidth limit in KiB/s.
- bandwidth_
limit_ intrestore - Restore I/O bandwidth limit in KiB/s.
- console str
- Select the default Console viewer. Must be
applet
|vv
|html5
|xtermjs
. You can either use the builtin java applet (VNC; deprecated and maps to html5), an external virt-viewer compatible application (SPICE), an HTML5 based vnc viewer (noVNC), or an HTML5 based console client (xtermjs). If the selected viewer is not available (e.g. SPICE not activated for the VM), the fallback is noVNC. - crs_
ha str - Cluster resource scheduling setting for HA. Must be
static
|basic
(default isbasic
). - crs_
ha_ boolrebalance_ on_ start - Cluster resource scheduling setting for HA rebalance on start.
- description str
- Datacenter description. Shown in the web-interface datacenter notes panel. This is saved as comment inside the configuration file.
- email_
from str - email address to send notification from (default is root@$hostname).
- ha_
shutdown_ strpolicy - Cluster wide HA shutdown policy (). Must be
freeze
|failover
|migrate
|conditional
(default isconditional
). - http_
proxy str - Specify external http proxy which is used for downloads (example:
http://username:password@host:port/
). - keyboard str
- Default keyboard layout for vnc server. Must be
de
|de-ch
|da
|en-gb
|en-us
|es
|fi
|fr
|fr-be
|fr-ca
|fr-ch
|hu
|is
|it
|ja
|lt
|mk
|nl
|no
|pl
|pt
|pt-br
|sv
|sl
|tr
. - language str
- Default GUI language. Must be
ca
|da
|de
|en
|es
|eu
|fa
|fr
|he
|it
|ja
|nb
|nn
|pl
|pt_BR
|ru
|sl
|sv
|tr
|zh_CN
|zh_TW
. - mac_
prefix str - Prefix for autogenerated MAC addresses.
- max_
workers int - Defines how many workers (per node) are maximal started on actions like 'stopall VMs' or task from the ha-manager.
- migration_
cidr str - Cluster wide migration network CIDR.
- migration_
type str - Cluster wide migration type. Must be
secure
|unsecure
(default issecure
). - next_
id cluster.Options Next Id Args - The ranges for the next free VM ID auto-selection pool.
- notify
cluster.
Options Notify Args - Cluster-wide notification settings.
- bandwidth
Limit NumberClone - Clone I/O bandwidth limit in KiB/s.
- bandwidth
Limit NumberDefault - Default I/O bandwidth limit in KiB/s.
- bandwidth
Limit NumberMigration - Migration I/O bandwidth limit in KiB/s.
- bandwidth
Limit NumberMove - Move I/O bandwidth limit in KiB/s.
- bandwidth
Limit NumberRestore - Restore I/O bandwidth limit in KiB/s.
- console String
- Select the default Console viewer. Must be
applet
|vv
|html5
|xtermjs
. You can either use the builtin java applet (VNC; deprecated and maps to html5), an external virt-viewer compatible application (SPICE), an HTML5 based vnc viewer (noVNC), or an HTML5 based console client (xtermjs). If the selected viewer is not available (e.g. SPICE not activated for the VM), the fallback is noVNC. - crs
Ha String - Cluster resource scheduling setting for HA. Must be
static
|basic
(default isbasic
). - Boolean
- Cluster resource scheduling setting for HA rebalance on start.
- description String
- Datacenter description. Shown in the web-interface datacenter notes panel. This is saved as comment inside the configuration file.
- email
From String - email address to send notification from (default is root@$hostname).
- ha
Shutdown StringPolicy - Cluster wide HA shutdown policy (). Must be
freeze
|failover
|migrate
|conditional
(default isconditional
). - http
Proxy String - Specify external http proxy which is used for downloads (example:
http://username:password@host:port/
). - keyboard String
- Default keyboard layout for vnc server. Must be
de
|de-ch
|da
|en-gb
|en-us
|es
|fi
|fr
|fr-be
|fr-ca
|fr-ch
|hu
|is
|it
|ja
|lt
|mk
|nl
|no
|pl
|pt
|pt-br
|sv
|sl
|tr
. - language String
- Default GUI language. Must be
ca
|da
|de
|en
|es
|eu
|fa
|fr
|he
|it
|ja
|nb
|nn
|pl
|pt_BR
|ru
|sl
|sv
|tr
|zh_CN
|zh_TW
. - mac
Prefix String - Prefix for autogenerated MAC addresses.
- max
Workers Number - Defines how many workers (per node) are maximal started on actions like 'stopall VMs' or task from the ha-manager.
- migration
Cidr String - Cluster wide migration network CIDR.
- migration
Type String - Cluster wide migration type. Must be
secure
|unsecure
(default issecure
). - next
Id Property Map - The ranges for the next free VM ID auto-selection pool.
- notify Property Map
- Cluster-wide notification settings.
Supporting Types
OptionsNextId, OptionsNextIdArgs
OptionsNotify, OptionsNotifyArgs
- Ha
Fencing stringMode - Cluster-wide notification settings for the HA fencing mode. Must be
always
|never
. - Ha
Fencing stringTarget - Cluster-wide notification settings for the HA fencing target.
- Package
Updates string - Cluster-wide notification settings for package updates. Must be
auto
|always
|never
. - Package
Updates stringTarget - Cluster-wide notification settings for the package updates target.
- Replication string
- Cluster-wide notification settings for replication. Must be
always
|never
. - Replication
Target string - Cluster-wide notification settings for the replication target.
- Ha
Fencing stringMode - Cluster-wide notification settings for the HA fencing mode. Must be
always
|never
. - Ha
Fencing stringTarget - Cluster-wide notification settings for the HA fencing target.
- Package
Updates string - Cluster-wide notification settings for package updates. Must be
auto
|always
|never
. - Package
Updates stringTarget - Cluster-wide notification settings for the package updates target.
- Replication string
- Cluster-wide notification settings for replication. Must be
always
|never
. - Replication
Target string - Cluster-wide notification settings for the replication target.
- ha
Fencing StringMode - Cluster-wide notification settings for the HA fencing mode. Must be
always
|never
. - ha
Fencing StringTarget - Cluster-wide notification settings for the HA fencing target.
- package
Updates String - Cluster-wide notification settings for package updates. Must be
auto
|always
|never
. - package
Updates StringTarget - Cluster-wide notification settings for the package updates target.
- replication String
- Cluster-wide notification settings for replication. Must be
always
|never
. - replication
Target String - Cluster-wide notification settings for the replication target.
- ha
Fencing stringMode - Cluster-wide notification settings for the HA fencing mode. Must be
always
|never
. - ha
Fencing stringTarget - Cluster-wide notification settings for the HA fencing target.
- package
Updates string - Cluster-wide notification settings for package updates. Must be
auto
|always
|never
. - package
Updates stringTarget - Cluster-wide notification settings for the package updates target.
- replication string
- Cluster-wide notification settings for replication. Must be
always
|never
. - replication
Target string - Cluster-wide notification settings for the replication target.
- ha_
fencing_ strmode - Cluster-wide notification settings for the HA fencing mode. Must be
always
|never
. - ha_
fencing_ strtarget - Cluster-wide notification settings for the HA fencing target.
- package_
updates str - Cluster-wide notification settings for package updates. Must be
auto
|always
|never
. - package_
updates_ strtarget - Cluster-wide notification settings for the package updates target.
- replication str
- Cluster-wide notification settings for replication. Must be
always
|never
. - replication_
target str - Cluster-wide notification settings for the replication target.
- ha
Fencing StringMode - Cluster-wide notification settings for the HA fencing mode. Must be
always
|never
. - ha
Fencing StringTarget - Cluster-wide notification settings for the HA fencing target.
- package
Updates String - Cluster-wide notification settings for package updates. Must be
auto
|always
|never
. - package
Updates StringTarget - Cluster-wide notification settings for the package updates target.
- replication String
- Cluster-wide notification settings for replication. Must be
always
|never
. - replication
Target String - Cluster-wide notification settings for the replication target.
Import
#!/usr/bin/env sh
Cluster options are global and can be imported using e.g.:
$ pulumi import proxmoxve:Cluster/options:Options options cluster
To learn more about importing existing cloud resources, see Importing resources.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
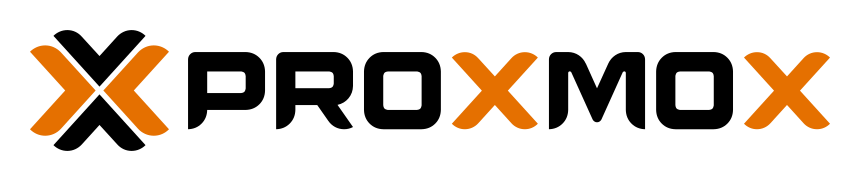