Proxmox Virtual Environment (Proxmox VE) v6.10.1 published on Friday, Jun 28, 2024 by Daniel Muehlbachler-Pietrzykowski
proxmoxve.Cluster.getNodes
Explore with Pulumi AI
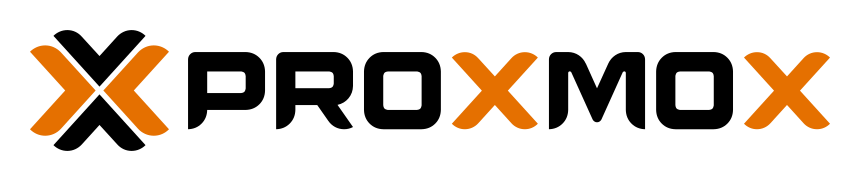
Proxmox Virtual Environment (Proxmox VE) v6.10.1 published on Friday, Jun 28, 2024 by Daniel Muehlbachler-Pietrzykowski
Retrieves information about all available nodes.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@pulumi/proxmoxve";
const availableNodes = proxmoxve.Cluster.getNodes({});
import pulumi
import pulumi_proxmoxve as proxmoxve
available_nodes = proxmoxve.Cluster.get_nodes()
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/Cluster"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := Cluster.GetNodes(ctx, nil, nil)
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var availableNodes = ProxmoxVE.Cluster.GetNodes.Invoke();
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Cluster.ClusterFunctions;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var availableNodes = ClusterFunctions.getNodes();
}
}
variables:
availableNodes:
fn::invoke:
Function: proxmoxve:Cluster:getNodes
Arguments: {}
Using getNodes
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getNodes(opts?: InvokeOptions): Promise<GetNodesResult>
function getNodesOutput(opts?: InvokeOptions): Output<GetNodesResult>
def get_nodes(opts: Optional[InvokeOptions] = None) -> GetNodesResult
def get_nodes_output(opts: Optional[InvokeOptions] = None) -> Output[GetNodesResult]
func GetNodes(ctx *Context, opts ...InvokeOption) (*GetNodesResult, error)
func GetNodesOutput(ctx *Context, opts ...InvokeOption) GetNodesResultOutput
> Note: This function is named GetNodes
in the Go SDK.
public static class GetNodes
{
public static Task<GetNodesResult> InvokeAsync(InvokeOptions? opts = null)
public static Output<GetNodesResult> Invoke(InvokeOptions? opts = null)
}
public static CompletableFuture<GetNodesResult> getNodes(InvokeOptions options)
// Output-based functions aren't available in Java yet
fn::invoke:
function: proxmoxve:Cluster/getNodes:getNodes
arguments:
# arguments dictionary
getNodes Result
The following output properties are available:
- Cpu
Counts List<int> - The CPU count for each node.
- Cpu
Utilizations List<double> - The CPU utilization on each node.
- Id string
- The provider-assigned unique ID for this managed resource.
- Memory
Availables List<int> - The memory available on each node.
- Memory
Useds List<int> - The memory used on each node.
- Names List<string>
- The node names.
- Onlines List<bool>
- Whether a node is online.
- Ssl
Fingerprints List<string> - The SSL fingerprint for each node.
- Support
Levels List<string> - The support level for each node.
- Uptimes List<int>
- The uptime in seconds for each node.
- Cpu
Counts []int - The CPU count for each node.
- Cpu
Utilizations []float64 - The CPU utilization on each node.
- Id string
- The provider-assigned unique ID for this managed resource.
- Memory
Availables []int - The memory available on each node.
- Memory
Useds []int - The memory used on each node.
- Names []string
- The node names.
- Onlines []bool
- Whether a node is online.
- Ssl
Fingerprints []string - The SSL fingerprint for each node.
- Support
Levels []string - The support level for each node.
- Uptimes []int
- The uptime in seconds for each node.
- cpu
Counts List<Integer> - The CPU count for each node.
- cpu
Utilizations List<Double> - The CPU utilization on each node.
- id String
- The provider-assigned unique ID for this managed resource.
- memory
Availables List<Integer> - The memory available on each node.
- memory
Useds List<Integer> - The memory used on each node.
- names List<String>
- The node names.
- onlines List<Boolean>
- Whether a node is online.
- ssl
Fingerprints List<String> - The SSL fingerprint for each node.
- support
Levels List<String> - The support level for each node.
- uptimes List<Integer>
- The uptime in seconds for each node.
- cpu
Counts number[] - The CPU count for each node.
- cpu
Utilizations number[] - The CPU utilization on each node.
- id string
- The provider-assigned unique ID for this managed resource.
- memory
Availables number[] - The memory available on each node.
- memory
Useds number[] - The memory used on each node.
- names string[]
- The node names.
- onlines boolean[]
- Whether a node is online.
- ssl
Fingerprints string[] - The SSL fingerprint for each node.
- support
Levels string[] - The support level for each node.
- uptimes number[]
- The uptime in seconds for each node.
- cpu_
counts Sequence[int] - The CPU count for each node.
- cpu_
utilizations Sequence[float] - The CPU utilization on each node.
- id str
- The provider-assigned unique ID for this managed resource.
- memory_
availables Sequence[int] - The memory available on each node.
- memory_
useds Sequence[int] - The memory used on each node.
- names Sequence[str]
- The node names.
- onlines Sequence[bool]
- Whether a node is online.
- ssl_
fingerprints Sequence[str] - The SSL fingerprint for each node.
- support_
levels Sequence[str] - The support level for each node.
- uptimes Sequence[int]
- The uptime in seconds for each node.
- cpu
Counts List<Number> - The CPU count for each node.
- cpu
Utilizations List<Number> - The CPU utilization on each node.
- id String
- The provider-assigned unique ID for this managed resource.
- memory
Availables List<Number> - The memory available on each node.
- memory
Useds List<Number> - The memory used on each node.
- names List<String>
- The node names.
- onlines List<Boolean>
- Whether a node is online.
- ssl
Fingerprints List<String> - The SSL fingerprint for each node.
- support
Levels List<String> - The support level for each node.
- uptimes List<Number>
- The uptime in seconds for each node.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
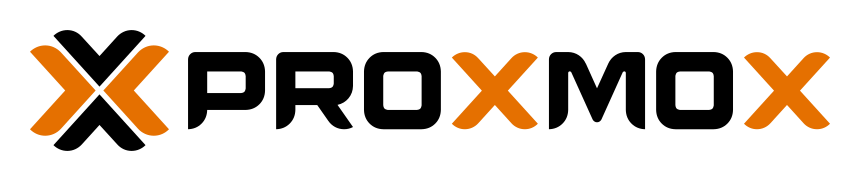
Proxmox Virtual Environment (Proxmox VE) v6.10.1 published on Friday, Jun 28, 2024 by Daniel Muehlbachler-Pietrzykowski